Vue Component for Inline Text Editing: Enhancing Web Interfaces
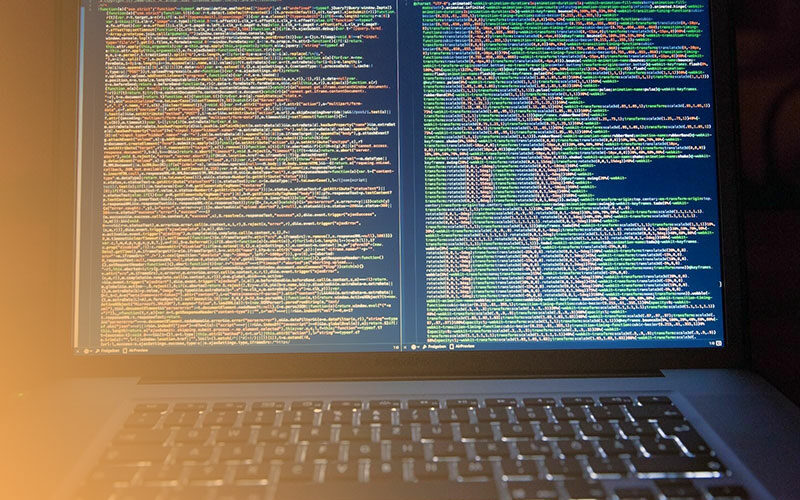
Do you ever wonder what would happen if you tried to write an entire essay using sticky notes? You probably don’t. But it would be something very inconvenient. This is pretty much the same level of inconvenience you’d feel when editing HTML directly. This applies to most end-users, not all. However, inline editing simplifies the long, tiresome process. How? By allowing you to see the edits live. What does that even mean? It means you can edit the text right where it appears on the web page.
So, who is to thank for such convenience? None other than WYSIWYG editors. The change from HTML to WYSIWYG has made editing pretty simple. It’s become easier for beginner developers to properly edit web pages without 5 long years of experience. In a way, the need to learn HTML code has almost entirely been eliminated. The Vue.js framework is ideal for this, thanks to its reactive data system and component-based approach.
​This article covers how Vue.js can make your life as a developer easier. How? By enhancing web interfaces with inline text editing. We’ll also have a look at the transition from HTML to WYSIWYG. And, of course, we’ll see how the use of Vue components in real-time editing improves the website’s UI/UX.
Understanding Vue Components
As developers, most of you already know about Vue.js. It’s a modern tool that has revolutionized web development. It lets developers build components of a website that can be used repeatedly. Yes, you read that right. These components are very independent. How so? Because they have their own set of instructions and design rules. This makes it easier to manage and update these individual parts of the site.
If you are new, here’s all you need to know about Vue. First of all, it uses a straightforward approach. You just have to lay out how things look using HTML templates. The Vue.js framework takes care of the rest. It also kicks in when there needs to be a change made. Vue updates the website on its own to reflect the new changes on the website. This is what you can refer to as “reactivity.” It keeps the app’s display in sync with its state.
Come to think of it, this setup is actually pretty solid for building text editors. You can just create one editor component and use it wherever you want on your site. Each editor works independently, so you can have multiple editors open at the same time without any issues.
Building an Inline Text Editor with Vue
​To build an inline text editor in Vue, you need to transform a static HTML element into a dynamic editing interface. Here are the steps you must take to do that:
- Bind a Static HTML Element to Vue: Start by binding a static HTML element, such as a ‘div’ or a ‘textarea’, to a Vue instance using the ‘v-model’ directive. This binds the content of the HTML element to a data property in your Vue component, making it reactive. For example:
<textarea v-model="text"></textarea>
Here, ‘text’ would be a data property in your Vue component that holds the content of the textarea.
- Toggle Between Edit and Display Modes: Add a button that allows users to switch between ‘edit’ and ‘display’ modes. Use Vue’s event handling to toggle a boolean data property when the button is clicked. Here’s an example:
<button @click="editMode = !editMode">Toggle Edit</button> <div v-if="editMode"> Â <textarea v-model="text"></textarea> </div> <div v-else> <p>{{ text }}</p> </div>
In this setup, ‘editMode’ is a boolean property that determines whether the textarea or a plain text paragraph is displayed.
- Integrate a WYSIWYG Library: If you’re not building the text editor from scratch, integrate a simple WYSIWYG library like Froala. Vue’s flexibility allows you to wrap these libraries in a Vue component. You can then control these libraries using Vue’s reactive data system, which can then be controlled using Vue’s reactive data system. For instance, after installing Froala:
import VueFroala from ‘vue-froala-wysiwyg’;
Vue.use(VueFroala);
Then, use it in your template by adding the Froala component wherever you need the rich text editor functionality.
<template> <div>   <froala :tag="'textarea'" :config="config" v-model="text"></froala> </div> </template> <script> import VueFroala from 'vue-froala-wysiwyg'; export default { name: 'App', components: {   VueFroala }, data() {   return {     text: 'Edit Your Content Here!', // Initial content for the editor     config: {       // Froala Editor options       toolbarButtons: ['bold', 'italic', 'underline', 'paragraphFormat'],       placeholderText: 'Edit Your Text Here!'     }   } } } </script>
As you can observe, the ‘<froala>’ component is used to create a rich text editor in the Vue application. The ‘v-model’ directive binds the editor’s content to the ‘text’ data property, making the editor’s content reactive. The ‘config’ object contains Froala-specific configurations, allowing customization of the toolbar and other features.
Enhancing User Experience with Vue
Vue directly enhances how smooth user interactions can be. Its reactivity system enables you to see updates on the screen in real-time. That’s a bonus in itself. Let’s understand this better with an example. If you update any product descriptions on an e-commerce platform, the changes will be made in the Vue-based WYSIWYG editor without any reloads on the page. The same goes for editing any comments on a blog.
This instant feedback and a pleasant user interface design are synonymous with a better user experience. Users feel engaged and happy that their input has been recorded instantly. As a developer, this is a major determinant of how successful your project has been. And Vue can be an excellent sidekick for you in achieving this.
Best Practices and Common Challenges
As a developer, you need to ensure readability and code maintainability. Maintaining a clean component structure directly relates to this. This can often get overwhelming when dealing with a WYSIWYG editor, but you should be fine as long as you follow the guide above. You also need to ensure that you have mechanisms in place to sanitize data and save any edited data. This will help protect against security threats, such as XSS.
The Vue WYSIWYG integration can be extremely rewarding. Naturally, it also brings its fair share of challenges. These issues include browser compatibility issues that result in different renditions of the edited content. Additionally, some features of WYSIWYG editors require more in-depth integration efforts to function across various platforms. These features include custom plugins and advanced formatting options.
Conclusion
Vue inline editors are key in converting HTML to WYSIWYG. This allows developers to utilize a seamless editing experience. Over the course of this guide, we have learned how Vue’s reactive nature and component-driven approach have improved user experience through real-time updates.
Looking ahead, there is a realm of possibilities thanks to Vue 3’s composition API. We’re looking at further possibilities for even more dynamic and flexible inline text editing solutions. So gear up and get ready to make the most of Vue’s offering for your development game.
FAQs
How does Vue.js improve inline text editing?
Vue.js updates edits in real-time and integrates easily with WYSIWYG editors for a seamless user experience.
What are the benefits of converting HTML to WYSIWYG in Vue?
It simplifies content editing, making it more accessible for non-technical users by eliminating the need to handle HTML code directly.
Can Vue components work with any WYSIWYG library?
Yes, Vue components can integrate with various WYSIWYG libraries, enhancing flexibility and editing capabilities within Vue apps.
- What Is Rust Used For? A Guide to Its Applications - May 4, 2024
- Simplifying Video Editing: Creative Apps Like Kapwing - May 3, 2024
- Healthcare software: Exploring 10 essential types and their benefits - May 3, 2024