Exploring the JavaScript While Loop for Repetitive Tasks
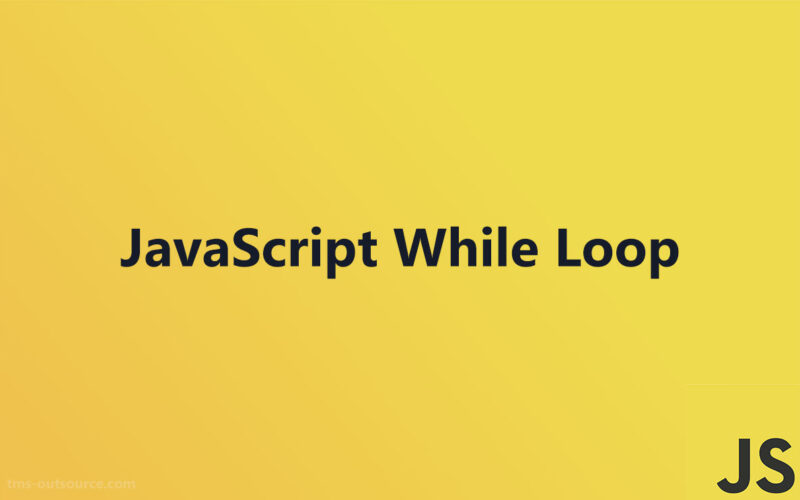
In the dynamic world of web development, mastering the JavaScript While Loop is crucial for any developer looking to enhance their coding efficiency.
This article delves into the syntax, execution, and practical applications of while loops, providing insights into optimizing code performance and avoiding common pitfalls.
With clear examples and best practices, you’ll gain the knowledge to implement these loops confidently in your projects.
Syntax and Basic Concepts
Structure of the While Loop
Understanding the structure of the JavaScript While Loop begins with grasping its syntax and how it functions during code execution.
The beauty of the while loop lies in its simplicity and flexibility, enabling developers to execute code blocks based on specified Boolean conditions.
Syntax overview from MDN
The syntax for a while loop, as outlined by Mozilla Developer Network (MDN), is straightforward. Here’s the basic form:
while (condition) {
// code block to be executed
}
In this construct, the condition
is evaluated before the execution of the loop’s code block. If the condition evaluates to true, the entered code executes. This process repeats until the condition returns false.
Execution flow described by W3Schools
According to W3Schools, the execution flow of a while loop checks the condition before entering into the loop body.
This pre-check ensures that if the condition is never true, the code inside the loop does not execute at all, preventing any unintended infinite loop scenarios.
This characteristic is essential for optimizing loop performance and ensuring efficient code execution in various application scenarios, such as data processing or managing user interaction within web applications.
The Do While Loop
Switching focus to the Do While Loop, which serves as a slight variation but with a crucial difference in terms of execution flow, which can be better for certain JavaScript development tools scenarios.
Syntax and basic examples from TutorialsPoint
TutorialsPoint provides a clear syntax and practical examples for understanding the Do While loop.
The general syntax appears as follows:
do {
// code block to execute
} while (condition);
This syntax executes the code block once before checking the condition at the end of the loop.
This trait guarantees that the loop’s body executes at least once, which can be particularly advantageous in situations where the initial iteration needs to run regardless of the condition’s state.
Key differences in execution flow from the While Loop
The key difference between the While and the Do While loops lies in when the condition is evaluated.
In a Do While loop, the condition is checked after the code block has executed, ensuring at least one iteration occurs.
This contrasts with the While loop, where the condition is evaluated before the code block executes.
This structural difference can significantly impact how and when certain code is executed within a program, especially when dealing with user inputs or processing initial data where at least one iteration is required for appropriate behavior or setup.
This understanding of syntax and basic operation between the While and Do While loops provides foundational knowledge necessary for implementing efficient looping mechanisms and fulfilling various JavaScript loop array or control flow requirements efficiently and effectively.
Working with While Loops
Practical Examples
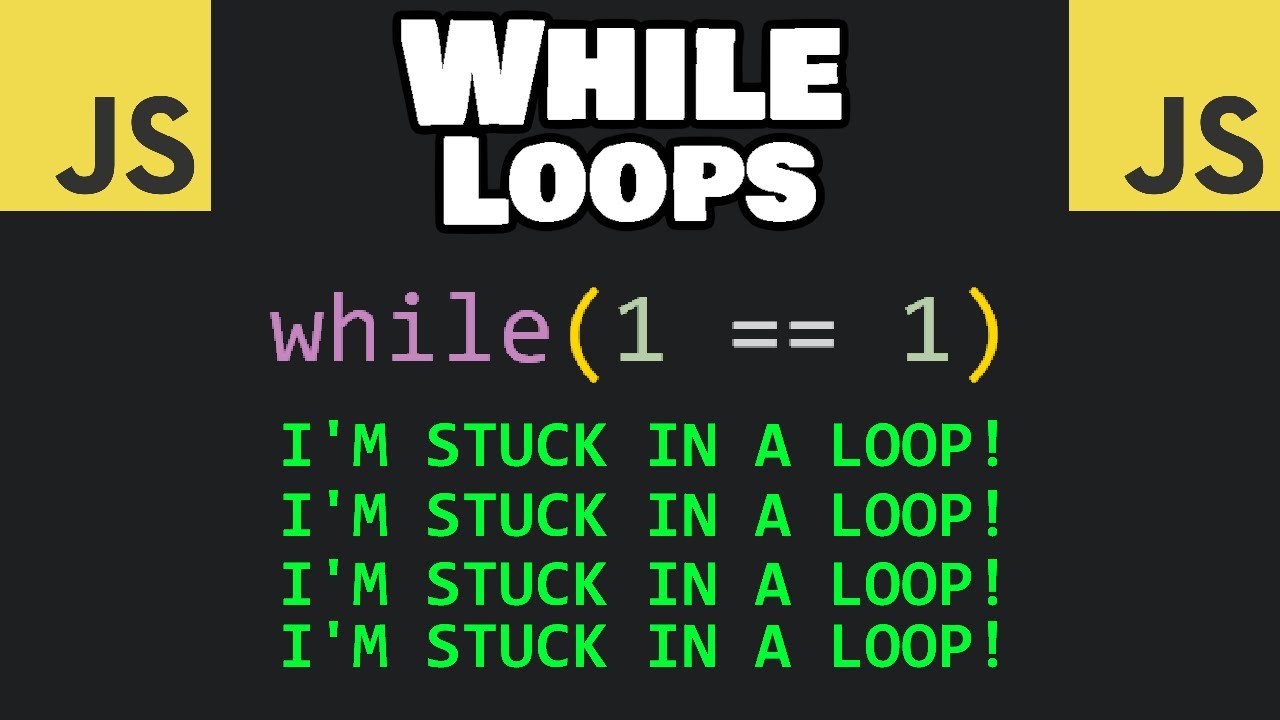
Let’s dive into some concrete situations where you might use a JavaScript While Loop or its variations.
These scenarios can range from basic iterations like counting, to more complex data manipulation with nested loops.
Simple counting loops
Consider a scenario where you need to execute a block of code five times. A simple while loop is efficient for this:
let count = 1;
while (count <= 5) {
console.log('Iteration number: ' + count);
count++;
}
This loop continues to execute as long as the condition (count <= 5
) is true, making it a great tool for handling basic counting mechanisms.
Nested loops and practical implications
Nested loops, a loop within another loop, are particularly useful for working with multi-dimensional arrays or complex data structures.
For instance, if you’re trying to find a particular value within a matrix (an array of arrays), nested loops can handle this efficiently:
let matrix = [[1, 2, 3], [4, 5, 6], [7, 8, 9]];
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
if (matrix[i][j] === 5) {
console.log('Found 5 at position: (' + i + ',' + j + ')');
}
}
}
While nested loops offer powerful functionality for handling complex data, they also come with the need for careful management to avoid performance issues such as slow execution times.
Control Flow within Loops
Managing how a loop executes is crucial for ensuring they perform effectively and avoid common errors like infinite loops.
Use of break and continue statements
In looping mechanisms, break
and continue
statements are essential for fine-tuning execution flow. The break
statement exits the loop immediately when a certain condition is met:
while (true) {
let userResponse = prompt("Enter 'exit' to stop:");
if (userResponse === 'exit') {
break;
}
console.log('You typed: ' + userResponse);
}
On the other hand, the continue
statement skips the current loop iteration and proceeds to the next one if a specific condition occurs:
while (condition) {
if (skipCondition) {
continue;
}
// Execute code if skipCondition is not met
}
These controls are particularly handy in real-world applications where decisions to either exit loops or skip iterations need to be made based on dynamic conditions.
Managing loop execution and avoiding infinite loops
Avoiding infinite loops, where a loop continues indefinitely without a stopping condition, is critical.
Ensuring that the condition in a while loop will eventually become false, either by modifying an external variable or through loop constructs, helps prevent such issues:
let start = 1;
while (start < 10) {
console.log(start);
start++; // Increment to ensure the loop condition will eventually be false
}
Carefully managing conditions and implementing proper exit strategies within loops ensures robust and error-free code execution in dynamic scenarios such as web development or software tutorials, enhancing both performance and user experience.
Detailed Examination of Do While Loops
Structure and Execution
Exploring the Do While loop offers insights not just into its syntax but also its unique execution flow, which ensures that the loop body executes at least once regardless of the condition at the beginning.
Syntax specifics from GeeksforGeeks
GeeksforGeeks provides a detailed look at the syntax of a Do While loop. Here’s how it’s generally structured:
do {
// Code to execute
} while (condition);
This shows that the code inside the do block executes first, and then the condition is checked. If the condition evaluates to true, the loop reruns. This sequence continues until the condition evaluates to false.
Guaranteed execution of loop body at least once
This characteristic is crucial for scenarios where you want to ensure that a set of operations executes at least once before a condition might stop further iterations.
This feature is especially useful in processing user input or managing initial setup tasks within a loop.
Practical Use Cases
Understanding when to use a Do While loop over a standard JavaScript While Loop can significantly enhance effectiveness in handling particular program flows or in iterating over programming syntax.
Appropriate scenarios for using Do While over While
The Do While loop is particularly advantageous in scenarios where the initial iteration needs to execute without conditional checks.
For instance, in user input validation where you must prompt at least once but continue only if the input does not meet certain criteria:
do {
var response = prompt("Enter your age (must be 18 or older to proceed):");
} while (response < 18);
This ensures the prompt displays at least once, providing a more user-friendly method for gathering valid inputs.
Example codes demonstrating the Do While Loop
Let’s look at a practical example demonstrating the use of Do While loops:
let result = '';
do {
result += "The number is " + i;
i++;
} while (i < 5);
console.log(result);
In this example, the loop compiles a string that lists numbers. The sequence always starts at “The number is 0” and continues until “The number is 4”, demonstrating how each loop iteration runs successfully before the condition is tested.
Understanding these nuances of Do While loops helps in precise and effective application, especially in tasks requiring at least one execution of the loop body, such as initializing scripts or creating recursive user interfaces in browser-based or software development environments.
Comparisons and Use Case Scenarios
Comparing While and Do While Loops
It’s important to grasp when to leverage one loop over another to enhance code efficiency and readability.
Both While and Do While loops serve crucial, though slightly different purposes in coding scenarios.
Entry vs. exit condition loops
The fundamental difference between these two loops lies in where the condition is evaluated.
In a JavaScript While Loop, the condition is evaluated at the entry point, which means the code within the loop may not execute at all if the condition is false from the start.
On the other hand, a Do While loop checks the condition at the exit point, ensuring that the code within the loop executes at least once before the condition is tested.
This guarantees at least a single run-through, regardless of the condition’s truth value—perfect for scenarios where initial execution is crucial.
Advantages and typical use cases for each
While loops are excellent when you need continuous checking of a condition from the get-go, such as waiting for a specific input to move forward with the process.
Do While loops shine in scenarios where an action must execute at least once and subsequent iterations depend on variable conditions obtained from the initial run. This makes them ideal for tasks like menu systems, where at least one prompt display is requisite.
While Loops vs. For Loops
Both While loops and For loops are central in handling repeated tasks, but choosing the right one depends on the specific needs of your application.
Structural differences and similar use cases
A For loop is typically used when the number of iterations is known before the loop starts, as it lets you initialize a counter, set a loop condition, and increment/decrement a counter all in one line:
for (let i = 0; i < 10; i++) {
// Execution block
}
While loops, conversely, are preferable when the number of iterations isn’t known beforehand, perhaps awaiting a certain condition to change during the loop’s execution, typically controlled externally by the user or some asynchronous operations.
Choosing the right loop for a given problem
Selecting the appropriate loop often depends on the structure and requirements of the specific problem.
Use a For loop when dealing with a fixed number of operations like iterating over arrays or running a counter-controlled iteration.
On the other hand, a While loop excels in situations where the continuation condition involves real-time data or user interactions, and the end of the loop is not predetermined but reliant on during-looped operations and conditions.
Each loop type offers distinct advantages for both simple and complex coding solutions, aiding in efficient algorithm design and easy-to-maintain code.
Choosing wisely between them often boils down to understanding the exact needs of your execution flow—from guaranteed executions to conditional looping, ensuring maximum code efficiency and smooth user experiences.
Advanced Concepts and Techniques
Performance Considerations
When employing JavaScript While Loop structures in software development, understanding their efficiency implications is vital for optimizing performance.
Efficiency of While Loops in JavaScript
While loops, due to their nature of checking the condition at the start of each iteration, can be quite efficient if the conditions are met quickly.
However, their performance can degrade if the condition resolution is computationally expensive or if the loop body is heavy.
Optimizing the condition’s evaluation and minimizing the code inside the loop can lead to more robust applications.
Impact of loop structure on performance
The way a loop is structured can significantly impact the overall efficiency of a JavaScript application.
For instance, deeply nested loops or loops that include heavy functions and procedures can drastically slow down execution speed.
Streamlining loop logic and avoiding unnecessary complexity within loops are crucial steps in enhancing performance.
Common Mistakes and Best Practices
Even for experienced developers, certain pitfalls in loop constructs can lead to issues like reduced performance and unintended infinite loops.
Typical errors in loop constructs
Some common mistakes include:
- Misplaced loop controls: such as incorrectly placing increment/decrement operators, leading to infinite or zero iterations.
- Improperly evaluated conditions: which could cause the loop never to execute or to execute indefinitely.
- Overloading the loop body: by including excessive logic or heavy computations that should rather be handled outside the loop.
Best practices for writing clean and efficient loops
To ensure that your loops are both clean and efficient, consider the following guidelines:
- Keep it simple: Focus on minimal logic within the loop body to improve readability and performance.
- Precise conditions: Make sure the loop’s condition is as direct and simple as possible to avoid unnecessary complexity and computation.
- Use appropriate loop types: Select the right loop for the job; while a while loop is great for many tasks, in some cases, a for loop might offer better performance with its compact form.
- Avoid side effects: Ensure that loop operations do not unintentionally impact other parts of the application by modifying shared or global state.
By adhering to these practices and actively avoiding the typical errors, developers can craft loops that are efficient, understandable, and maintain optimal performance in larger applications.
FAQ On JavaScript While Loop
What is a JavaScript While Loop?
A JavaScript While Loop repeatedly executes a block of code as long as a specified condition remains true. It’s a fundamental tool for tasks that require repetitive actions, like iterating through arrays or managing timers in web development scenarios.
How do you write a basic While Loop in JavaScript?
To craft a basic While Loop, you initialize a condition before the loop starts, and then enclose the code you want to repeatedly execute within curly braces. Here is the typical syntax:
while (condition) {
// code to execute
}
What distinguishes a While Loop from a Do While Loop?
In a While Loop, the condition is evaluated before the code within the loop is executed. Contrast this with a Do While Loop, where the code block executes once before the condition is checked. This guarantees at least one execution of the loop body.
When should I use a Do While Loop instead of a While Loop?
Opt for a Do While Loop when you need to ensure the code within the loop executes at least once, regardless of whether the loop condition is initially true. It’s ideal for cases such as prompting user input that requires validation before proceeding.
Can a JavaScript While Loop become infinite?
Yes, if the loop condition never resolves to false, the While Loop will continue indefinitely, potentially causing the browser to freeze or your application to crash. Leveraging break statements judiciously can prevent such issues.
What are common mistakes made with While Loops?
A typical error is creating an infinite loop by not modifying the loop condition within the loop body or through a break condition. Others include overcomplicating the condition check or insufficiently planning for how the loop exits.
How can I exit a JavaScript While Loop early?
You can exit a While Loop early using a break statement. Placing break
within the loop’s body will immediately terminate the loop when triggered, regardless of the loop condition.
while (true) {
if (needToExit) break;
}
What is the best practice for optimizing a While Loop?
For optimal performance, keep the loop condition simple and avoid complex calculations within it. Regularly assess whether the condition may benefit from being more concise or if other loop constructs could be more suitable based on the task. This ensures faster and more efficient loop execution.
How do While Loops impact performance in JavaScript applications?
While Loops can strain application performance, especially if they execute heavy tasks or complex conditions repetitively. To mitigate potential slowdowns, optimize the condition and ensure necessary actions within the loop are as efficient as possible, balancing workload and execution times skillfully.
Can you nest a While Loop inside another loop?
Absolutely, nesting While Loops is possible and sometimes necessary to handle multi-dimensional data or complex tasks. However, beware of the increased risk of performance penalties and the complexity added to your code.
It’s vital to keep each loop’s logic clear and maintain performance by minimizing the operations performed within the inner loop.
Conclusion
In summation, the JavaScript While Loop stands as an irreplaceable construct within the programming landscape, perfectly tailored for scenarios demanding repetitive task execution until a specified condition changes.
Whether navigating through arrays, managing time-sensitive operations, or creating interactive user experiences, mastering this loop is pivotal. Remember, simplicity in your condition checks and loop control can lead to cleaner and more efficient code.
Embrace the power of the While Loop, and use it to enhance the dynamism and responsiveness of your web applications, ensuring you deliver a seamless user experience every time.
If you liked this article about JavaScript While Loop, you should check out this article about JavaScript Events.
There are also similar articles discussing external javascript, javascript loops, JavaScript For Loop, and JavaScript for-in Loop.
And let’s not forget about articles on JavaScript For Of, JavaScript do…while Loop, javascript foreach loop, and JavaScript Versions.
- Essentials of JavaScript Functions for Beginners - June 22, 2024
- Exclusive Hip-Hop Mixes: Music Apps Like Datpiff - June 21, 2024
- Revolutionizing Software Testing: How AI is Transforming Quality Assurance - June 21, 2024