A Beginner’s Guide to JavaScript Versions
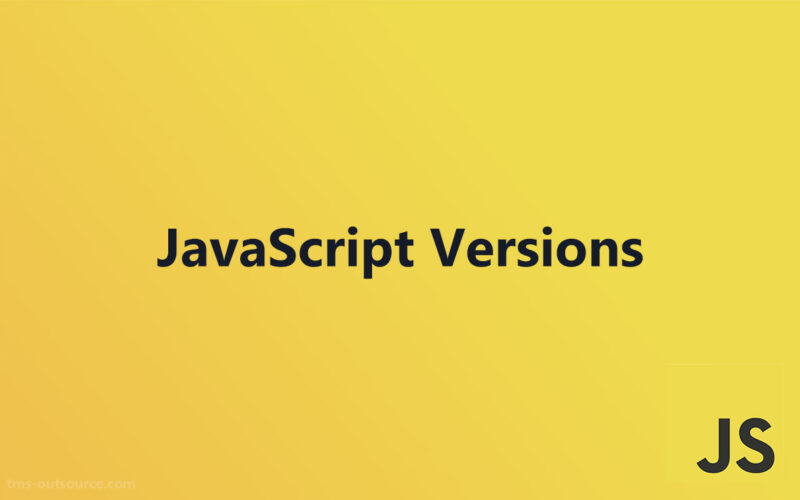
Exploring the evolution of JavaScript Versions reveals a dynamic history of updates designed to enhance coding efficiency and capabilities.
From its humble beginnings to powerful modern iterations like ECMAScript 2023 (ES14), each version has contributed significantly to web development practices.
This article delves into the nuances of each significant release and the impact theyāve had on the programming landscape.
JavaScript Versions
Version | Release Date | Key Features | Notable Improvements | Compatibility |
---|---|---|---|---|
ES3 | December 1999 | Regular expressions, try/catch | Error handling introduced | Widely supported in all browsers |
ES5 | December 2009 | Strict mode, JSON support, Array methods | Improved performance, better standards | Supported by all modern browsers |
ES6 (ES2015) | June 2015 | Let/const, Arrow functions, Promises, Classes | Significant language enhancements | Supported by most modern browsers |
ES7 (ES2016) | June 2016 | Exponentiation operator, Array.prototype.includes | Small updates with powerful features | Supported by modern browsers |
ES8 (ES2017) | June 2017 | Async/Await, Object.values(), Object.entries() | Simplified asynchronous code | Supported by most modern browsers |
ES9 (ES2018) | June 2018 | Asynchronous iteration, Rest/Spread properties | Enhanced object manipulation and async handling | Supported by modern browsers |
ES10 (ES2019) | June 2019 | Array.flat(), Array.flatMap(), Object.fromEntries() | Improved array handling and object conversion | Supported by modern browsers |
ES11 (ES2020) | June 2020 | BigInt, Dynamic Import, Nullish Coalescing | Improved numerical and modular capabilities | Supported by modern browsers |
ES12 (ES2021) | June 2021 | Logical assignment operators, WeakRefs | Enhanced memory management | Supported by modern browsers |
ES13 (ES2022) | June 2022 | Top-level await, RegExp match indices | Enhanced asynchronous programming | Supported by modern browsers |
ES14 (ES2023) | June 2023 | Array grouping, Decorators | Improved data manipulation, enhanced syntax | Supported by modern browsers |
Early JavaScript Versions
ECMAScript 1 (1997)
Key Features
The launch of ECMAScript 1 marked a pivotal moment in web development, introducing a standardized scripting language that would eventually become known as JavaScript.
Key features of this initial version included basic elements like variables, types, and operators, which laid the groundwork for more complex interactions on web pages.
Impact on Web Development
The introduction of ECMAScript significantly shaped web development practices.
It enabled more dynamic and interactive web experiences, moving away from static HTML pages.
This shift allowed developers to create richer user interfaces and improve user engagement across web platforms.
ECMAScript 2 (1998)
Minor Changes
ECMAScript 2 brought minimal functional changes to the language.
The focus was more on aligning with the ISO/IEC 16262 standard, which included minor syntactical adjustments to enhance readability and consistency in coding practices.
Standardization Improvements
More importantly, the alignment with international standards ensured that ECMAScript and hence JavaScript could be reliably used in a broader range of devices and environments, a crucial step for ensuring cross-browser compatibility.
ECMAScript 3 (1999)
Major Enhancements
1999’s ECMAScript 3 introduced several major enhancements that greatly extended the language’s capabilities.
This version included more robust error handling with try/catch statements, better string handling, and more versatile options for formatting numbers.
Introduction of Regular Expressions
Regular expressions, introduced in this version, were a game-changer for JavaScript.
They allowed for advanced search and text manipulation capabilities within web applications, making JavaScript an even more powerful tool for web developers.
New Control Structures
The addition of new control structures, such as switch statements and do-while loops, provided developers with more flexibility and control over the flow of JavaScript code.
This allowed for more complex, efficient, and readable scriptsāvital for developing advanced web applications.
Transitional Phase
ECMAScript 4 (2000, Never Released)
Proposed Features
ECMAScript 4 aimed to introduce a robust set of features that could significantly enhance scripting capabilities and development practices.
These proposed features included class-based object-oriented programming, optional type annotations, and improved namespace control, setting the stage for what could have been a transformative update.
Reasons for Non-Release
Despite the ambitious vision, ECMAScript 4 was never released. The primary reasons were concerns over the complexity it might introduce and the significant changes required in existing implementations.
There was also a marked division within the community and amongst various stakeholders, including major tech companies, about the direction of these proposed updates.
ECMAScript 5 (2009)
Strict Mode
One of the key features introduced in ECMAScript 5 was “Strict Mode.” This is a subset of JavaScript that doesn’t allow certain syntax to prevent common coding bugs and issues.
By opting into strict mode, developers can avoid problematic scenarios such as inadvertently creating global variables or deletions of undeletable properties, thus fostering a cleaner and more error-resilient coding environment.
JSON Support
ECMAScript 5 added built-in native JSON support, streamlining data interchange between servers and clients.
JSON parsing and stringification became inherently faster and more secure, owing to the elimination of the need for third-party libraries which could sometimes lead to security vulnerabilities.
Property Accessors
The introduction of getter and setter functions as part of property accessors in ECMAScript 5 allowed enhanced control over how properties are accessed and modified within objects.
This feature enabled encapsulation and more sophisticated object behaviors, facilitating fine-grained control over data with object literal enhancements.
Modern JavaScript Versions
ECMAScript 5.1 (2011)
Minor Revisions
ECMAScript 5.1 was essentially a maintenance update to iron out inconsistencies and ambiguities found in the original ECMAScript 5 specification.
It focused on clarifying parts of the specification and making minor adjustments to align with practical implementations that were already occurring in browsers.
Compliance with ISO/IEC 16262:2011
This update also marked ECMAScriptās full alignment with the international standard, ISO/IEC 16262:2011.
This compliance was crucial for ensuring that JavaScript remained a reliable and universally acceptable language across different software platforms.
ECMAScript 2015 (6th Edition)
Introduction of let and const
The introduction ofĀ let
Ā andĀ const
Ā for variable declarations was a significant shift, providing developers with more control over variable scoping by limiting their visibility to the block level, unlikeĀ var
Ā which is function-scoped.
Arrow Functions
Arrow functions not only provided a more concise syntax but also mitigated common pitfalls associated with theĀ this
Ā keyword in traditional JavaScript functions.
They are particularly useful in functional programming patterns and asynchronous JavaScript code.
Classes and Modules
ECMAScript 2015 formally introduced classes and modules, facilitating a more structured approach to JavaScript coding, akin to classical object-oriented languages.
This allowed for better code organization and reuse, which was a significant enhancement for large-scale application development.
Promises and Iterators
This edition also standardized Promises, representing a monumental leap forward in handling asynchronous operations.
Iterators and for…of loops were introduced, improving the way sequences and collections are iterated.
ECMAScript 2016 (7th Edition)
Exponentiation Operator
The exponentiation operatorĀ **
, a syntactic convenience, was added, allowing developers to perform exponential calculations without callingĀ Math.pow()
.
This small yet practical addition simplified many algorithms involving mathematical calculations.
Array.prototype.includes
Finally,Ā Array.prototype.includes
Ā method was introduced, making it much easier to determine if a certain value exists within an array.
This method provided a more readable alternative toĀ indexOf()
Ā and helped in writing clearer and more intuitive array-handling code.
Recent JavaScript Versions
ECMAScript 2017 (8th Edition)
Async/Await
Introduced to simplify writing asynchronous code, async/await allows developers to write promise-based code as if it were synchronous, but without blocking the execution thread.
This leads to cleaner, more readable code, especially when managing multiple asynchronous operations.
Shared Memory and Atomics
Shared memory and atomics provide developers with tools to manage memory accessibility between multiple threads and perform atomic operations. This is critical for developing high-performance, concurrent applications in JavaScript.
ECMAScript 2018 (9th Edition)
Asynchronous Iteration
This feature extends the async/await syntax and makes it possible to use with iterations, allowing theĀ for-await-of
Ā loop to iterate over asynchronous iterable objects like those returned by asynchronous generators.
Promise.finally()
Promise.finally() is a handler that is executed when the Promise is settled, regardless of its outcome.
It provides a way to execute cleanup code or final steps after a promise chain, without needing to duplicate code in both the promiseās fulfillment and rejection handlers.
ECMAScript 2019 (10th Edition)
Array.prototype.flat and flatMap
These methods simplify array manipulations:Ā flat()
Ā helps in flattening multi-layered arrays into a single-level array, whileĀ flatMap()
Ā combines mapping and flattening, streamlining the process of applying a function to each element and then flattening the result.
Object.fromEntries
Object.fromEntries
Ā transitions a list of key-value pairs into an object.
This is particularly useful when working with data transformed from arrays or other structures into objects.
ECMAScript 2020 (11th Edition)
BigInt
BigInt is a built-in object that provides a way to represent whole numbers larger than 2^53 – 1, which is the largest number JavaScript could reliably represent with the Number primitive.
Dynamic Import
Dynamic imports introduce a method to asynchronously request modules as needed, providing a way to reduce initial loading time by splitting the codebase into smaller chunks.
Nullish Coalescing Operator
The nullish coalescing operatorĀ ??
Ā provides a way to handle default values, specifically designed to work with cases where values might beĀ null
Ā orĀ undefined
, distinguishing these from falsy values like 0 or ”.
ECMAScript 2021 (12th Edition)
Logical Assignment Operators
Logical assignment operators combine logical operations with assignment, which can simplify certain assignments in code, such as usingĀ ||=
,Ā &&=
, andĀ ??=
Ā to assign values based on conditions more succinctly.
String.prototype.replaceAll
The replaceAll method allows string replacements to be performed across all instances within the string without needing to use a global regular expression.
ECMAScript 2022 (13th Edition)
Top-level await
Top-level await enables modules to act as asynchronous, allowing developers to use theĀ await
Ā keyword outside of async functions within modules.
This can simplify scripting in modules directly use dynamic imports or perform other asynchronous tasks before exporting.
Class Static Blocks
Class static blocks provide a way to perform additional static initializations during class definition.
This can be useful for cases where complex computations or asynchronous operations are needed before a class is used.
ECMAScript 2023 (14th Edition)
New Features Overview
The most recent updates include several performance optimizations and syntactical enhancements that continue to support modern development practices.
Impact on Development Practices
Each update to JavaScript versions ensures that the language evolves in lockstep with the changing landscape of web development, addressing both the needs for advanced functionality and the simplicity in coding practice.
Understanding ECMAScript Proposals
Proposal Stages
Stage 0: Strawman
The “Strawman” stage is where new ideas for JavaScript Versions are first presented.
At this level, an idea only needs to be identified as a potential problem or opportunity without thorough backing detail. It is primarily for discussing and gathering initial feedback.
Stage 1: Proposal
Once an idea has solidified into a tangible proposal, it graduates to Stage 1. Here, the proposer must clearly define the problem and demonstrate how their solution effectively addresses it.
The proposal also requires a champion, typically a member of the ECMAScript committee, who will advocate for the proposal through the subsequent stages.
Stage 2: Draft
At the “Draft” stage, the initial version of the proposal’s specification is written. Itās not complete, but enough for the committee to consider its feasibility.
The expectations are to shape the proposal into something that can be implemented, including precise syntax and semantics.
Stage 3: Candidate
By reaching the “Candidate” stage, the proposal is expected to have complete specification text.
The focus shifts to feedback from implementations and user experience to refine the proposal. Successful proposals at this stage are considered stable and undergo minimal changes.
Stage 4: Finished
A “Finished” proposal is one that has been accepted by the committee. It has been tested in practical applications and is ready to be included in the official ECMAScript specification.
Once a proposal reaches this stage, it is intended for inclusion in the next release of the specification.
Contribution Process
How Proposals Are Made
Proposals for new features or updates typically stem from developers, engineers, or other members of the JavaScript community.
Proposals must define a clear problem and a detailed solution with potential examples and polyfills. The process is meant to be open and organic, encouraging contributions that address genuine needs in the community.
Community and Committee Involvement
The ECMAScript committee, TC39, plays a crucial role, overseeing every stage of the proposal process.
This includes members from various organizations who bring diverse perspectives. However, the wider JavaScript community also contributes by providing feedback, participating in discussions, and experimenting with polyfills and shims.
This collaborative effort ensures that the language evolves in a way that benefits the whole ecosystem.
FAQ On JavaScript Versions
What is JavaScript used for in web development?
JavaScript breathes life into web pages.Ā It’s the magic that turns static HTML and CSS into interactive applications. From front-end logic to dynamic content updates, JavaScript is indispensable for creating responsive and engaging user interfaces that users love to interact with.
How does ECMAScript relate to JavaScript?
ECMAScript is the standard upon which JavaScript is built.Ā Think of ECMAScript as the blueprint that defines the language syntax, types, statements, and more.
JavaScript versions are implementations of various editions of the ECMAScript standard, ensuring that all adhere reliably to a unified set of guidelines.
What distinguishes JavaScript ES6 from previous versions?
ES6, or ECMAScript 2015, introduced groundbreaking features.Ā These included let and const for block scope, arrow functions for concise syntax, and promises for managing asynchronous operations. Each of these elevated JavaScript’s functionality, making coding more efficient and robust.
Can I use new JavaScript versions in older browsers?
Backward compatibility varies.Ā While new JavaScript versions bring exciting features, older browsers may not support them fully. To bridge this gap, developers often use transpilers (like Babel) to convert modern JavaScript into an older syntax that browsers can understand more universally.
What are the benefits of using the latest JavaScript versions?
Using the latest versions ensures access to the newest features and optimizations.Ā Modern JavaScript versions offer enhancements like improved security, better performance, and more intuitive syntax, which enhance web development efficiency and maintainability.
How often are new JavaScript versions released?
ECMAScript versions are now released annually.Ā This regular cadence was established to provide predictable and manageable updates. Each release usually includes a blend of new features and refinements to existing functionalities, aimed at continuously improving the language.
What is TypeScript and how is it related to JavaScript?
TypeScript is a superset of JavaScript.Ā It adds static types to the language, helping developers catch errors early and manage large codebases more effectively. TypeScript ultimately compiles down to JavaScript, making it a robust tool for development in complex architectures.
How can I check if a JavaScript feature is supported in my browser?
Use feature detection.Ā Libraries like Modernizr can help gauge whether specific features are available in a user’s browser. Additionally, checking the CanIUse website provides an up-to-date reference on browser support for various JavaScript features and APIs.
What are polyfills in the context of JavaScript?
Polyfills enable newer features to run in older environments.Ā They are pieces of code that provide modern functionality on older platforms that do not natively support it. This makes it possible to use the latest JavaScript features while maintaining compatibility across diverse browser landscapes.
Why was ECMAScript 4 never released?
ECMAScript 4 was too ambitious.Ā It proposed significant changes that created controversy among developers and companies due to the complexity and potential backward compatibility issues. Ultimately, it was abandoned in favor of more incremental, consensus-driven updates that led to ECMAScript 5.
Conclusion
Throughout this exploration of JavaScript Versions, it’s clear how each iteration of ECMAScript has contributed distinctly to the evolving web development landscape.
From enablingĀ dynamic user interactionsĀ to introducingĀ complex data handling with Arrays and Objects,Ā these updates continuously refine and enhance the capabilities of developers across the globe.
As the web grows more intricate, staying updated with these versions not only ensures compatibility but also leverages cutting-edge features that make modern web applications possible.
Ultimately, the journey through JavaScript’s evolution is a testament to the ever-adapting, innovative spirit of the web development community.
If you liked this article about JavaScript Versions, you should check out this article about JavaScript Events.
There are also similar articles discussing external javascript, javascript loops, JavaScript For Loop, and JavaScript While Loop.
And let’s not forget about articles on JavaScript for-in Loop, JavaScript For Of, JavaScript do…while Loop, and javascript foreach loop.
- Alternative App Stores: Discover Apps Like Aptoide - June 14, 2024
- How Are Desktop App, Web App, and Mobile App Similar and Different? - June 14, 2024
- Mastering JavaScript Logical Operators for Effective Logic - June 14, 2024