Mastering JavaScript Logical Operators for Effective Logic

In the intricate world of coding, understanding JavaScript Logical Operators is crucial. These operators—AND, OR, and NOT—form the backbone of decision-making in JavaScript.
They help programmers efficiently control the flow of operations and manage conditions within their code, enhancing both functionality and user interaction. This introduction delves into their practical applications and essential nuances, ensuring a solid grasp for both beginners and experienced developers.
Understanding Comparison Operators
Basic Comparison Operators
When we dive into the world of JavaScript, understanding the fundamentals like the equality and inequality operators is crucial.
We start with the simplest forms: Equal to (==) and Not equal to (!=). These operators compare two values for equivalence in value, disregarding their type.
Moving a step further, we encounter Strict equality (===) and Strict inequality (!==). Unlike their more lenient counterparts, these operators also take the datatype into consideration, ensuring that both the value and type match, or do not match, respectively. This distinction is vital for reliable programming, ensuring that your applications behave as expected without type coercion unexpectedly altering results.
Relational Operators
Relational operators determine how two values relate to each other in a: greater than, less than, or equal context. The basic Greater than (>) and Less than (<) operators are straightforward — they check if one value is greater or smaller than the other.
The Greater than or equal to (>=) and Less than or equal to (<=) expand on this by including equality as a condition.
These are particularly useful in range checks and loops, where you need to ensure a value falls within a certain boundary, including the boundaries themselves.
Practical Usage of Comparison Operators
In practical scenarios, understanding and implementing comparison operators is essential for effective decision-making in code.
Whether you’re working on decision logic in if-else statements or determining the flow of execution, these operators are fundamental tools.
For instance, in form validation, comparison operators help ensure that inputs like age, quantities, or sizes meet specific criteria before processing.
If the user input does not satisfy the conditions, feedback can be delivered promptly, enhancing user experience and maintaining data integrity.
Similarly, in managing control flow, comparison operators dictate how your program behaves under different conditions, guiding the execution paths.
This is evident in features like feature toggles, where you assess whether certain functionalities should be available to a user based on specific criteria or JavaScript logical operators.
By understanding how to use these operators, developers can write cleaner, more efficient code, boosting both performance and maintainability.
Detailed Look at Logical Operators
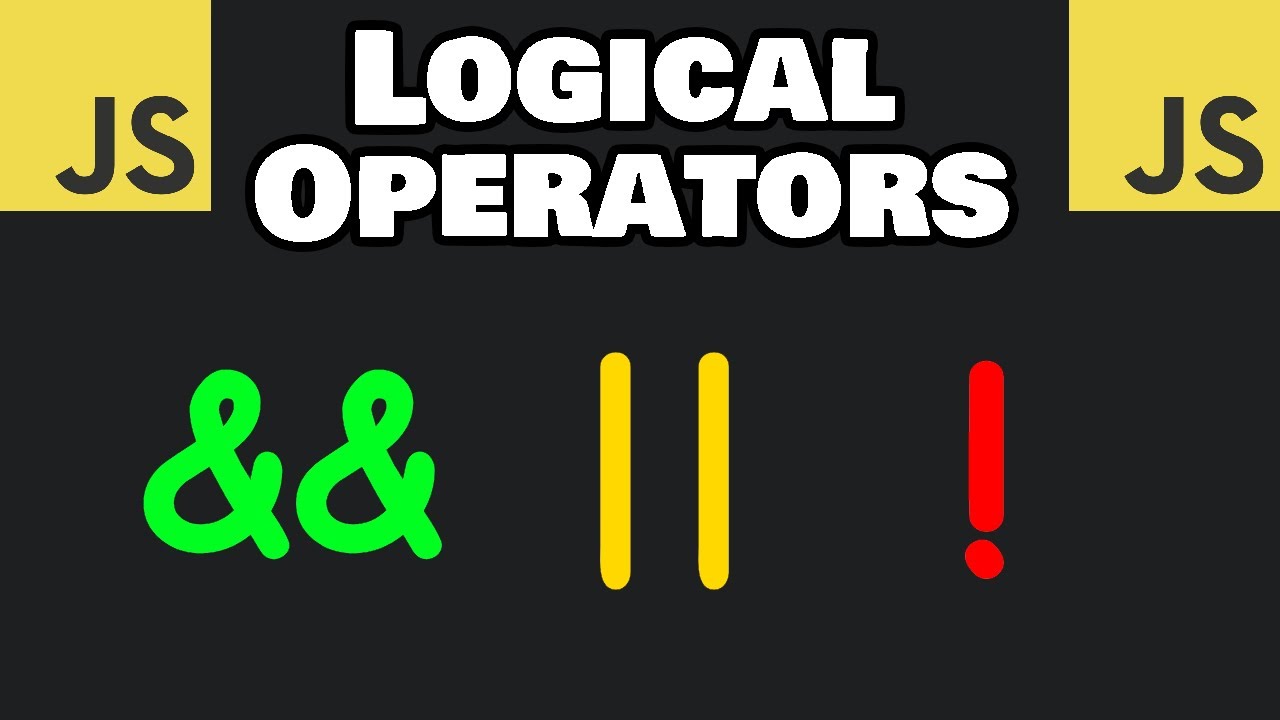
AND Operator (&&)
When discussing JavaScript Logical Operators, the AND operator (&&) is fundamental. It returns true only if all conditions being compared evaluate to true.
This characteristic makes it invaluable, especially in areas like user authentication and permission systems.
Consider a scenario where access is granted only if a user is logged in and has the correct permissions.
The AND operator ensures both conditions meet the required security checks, strengthening system integrity.
OR Operator (||)
Moving on to the OR operator (||), it takes a more lenient approach. This operator returns true if at least one of the conditions is true.
Its flexibility is particularly useful in settings where multiple conditions can lead to the same outcome.
In web development, OR is frequently used in feature toggles or to set default settings.
For instance, a feature might be enabled if it’s still in beta testing or if the user opts into beta features. It allows for more dynamic and user-friendly applications.
NOT Operator (!)
Lastly, the NOT operator (!) plays an essential role by inverting the truthiness of any expression.
It is a simple yet powerful tool for controlling the flow of conditions wherein the desired outcome is the opposite of a condition’s state.
Applications of the NOT operator are widespread, ranging from toggling boolean states to complex conditional rendering within UI frameworks.
It’s also a staple in error handling, where you might check if something hasn’t happened to throw an error or revert actions.
Advanced Operator Concepts
Short-Circuit Evaluation
Understanding short-circuit evaluation sheds light on how JavaScript Logical Operators optimize performance by skipping unnecessary computation.
The concept is straightforward: in logical expressions using AND (&&) or OR (||) operators, evaluation stops as soon as the outcome is determined.
For AND, this means if the first condition is false, there’s no need to check the second because the whole expression can never be true.
Similarly, for OR, if the first condition is true, the second isn’t evaluated because the expression is already true. This not only speeds up processing but also prevents potential side effects from executing unnecessary code.
Nullish Coalescing Operator (??)
The nullish coalescing operator (??) is a more precise tool than the logical OR for handling cases when values might be null or undefined.
It returns the right operand only when the left operand is nullish (null or undefined), not merely “falsy” like 0 or an empty string, which are considered valid by the logical OR.
In default assignment scenarios, this operator provides a more accurate method than OR, ensuring that defaults are only applied when values are truly absent, not merely falsy.
This clarity prevents common bugs in JavaScript applications, especially when dealing with optional object properties or configurations.
Optional Chaining Operator (?.)
Dealing with deeply nested objects can often lead to verbose and error-prone code.
The optional chaining operator (?.) introduces a more elegant solution. It allows you to safely access deeply nested properties without having to check if every part along the chain is valid.
If any part of the chain is undefined or null, the expression short-circuits and returns undefined, eliminating the risk of hitting a TypeError.
This operator is incredibly helpful in complex applications with layered data structures, simplifying interactions with potentially incomplete data and enhancing code robustness.
Applying Operators in JavaScript Code
Conditional Logic Implementation
Integrating multiple operators, such as deciding when and how to use JavaScript Logical Operators, plays a critical role in controlling the flow of decisions in programming.
This integration can be seen in scenarios where multiple conditions must align to execute a given block of code.
For example, consider a web application where access to certain features requires a user to be both a subscriber and an admin.
Here, logical AND (&&) helps ensure that both conditions are satisfied before granting access. By integrating various operators, developers can manage more complex conditional logic efficiently, ensuring that each component of the condition is clear and succinct.
Error Checking and Validation
Operators are indispensable tools in ensuring data integrity and validating user inputs. By using a combination of logical and comparison operators, developers can create thorough validation checks that improve the application’s security and reliability.
An example might involve ensuring that a numerical input falls within a specific range before it is processed.
Using comparison operators like greater than (>) and less than (<) along with logical OR (||) allows the program to provide immediate feedback if the input is invalid. This use of operators ensures that only appropriate and expected data is processed, protecting the application from potential bugs and security vulnerabilities.
Enhancing Code Readability and Maintenance
The clarity of code is fundamental, not just for current development but for future maintenance. Utilizing operators effectively can significantly enhance code readability, making it easier for others (or oneself at a later date) to understand and manage the code.
Best practices include using clear and concise logic with operators to keep the codebase manageable. Additionally, for older or legacy JavaScript code, refactoring to incorporate updated operator usage can reduce complexity and improve performance.
This might involve replacing outdated methods with newer, more efficient constructs like the optional chaining operator (?.), which can streamline code by reducing the need for repeated null checks and making the code cleaner and more intuitive.
Operators in Practice
Real-world Applications
Operators, especially JavaScript Logical Operators, significantly influence the structure and effectiveness of JavaScript frameworks. For instance, consider how AngularJS uses these operators in its two-way data binding and scope manipulation.
By efficiently controlling application states based on user inputs and interactions, these operators enhance both performance and responsiveness.
Similarly, in React, conditional rendering practices heavily depend on logical operators to manage dynamic content display.
This not only optimizes performance but also ensures that the user experience remains smooth and intuitive, as only necessary components re-render based on state changes.
Common Pitfalls and How to Avoid Them
Even experienced developers can sometimes trip over the nuances of using JavaScript operators. A common error includes misunderstandings around type coercion with the double equals (==) and not using the strict equality (===) when the situation demands preciseness in value and type.
To mitigate these issues, employing debugging tools such as Chrome DevTools, which provides insights and breakpoints to test how operators handle data during runtime, can be invaluable.
Adopting consistent coding standards that favor explicit over implicit comparisons is another way to reduce bugs related to unexpected type coercion and logic errors.
FAQ On JavaScript Logical Operators
What exactly are JavaScript Logical Operators?
Logical Operators in JavaScript assist in decision-making between two or more conditions. They are essential blocks in developing dynamic applications where outcomes change based on varying user inputs or other conditions.
How do the AND, OR, and NOT operators function?
The AND operator (&&) checks if all conditions are true, OR (||) validates if at least one condition is true, and NOT (!) reverses the truthiness of a condition. Each plays a pivotal role in controlling program flow and making decisions in code.
Can you nest JavaScript Logical Operators in conditions?
Absolutely, nesting logical operators allows for evaluating complex conditions by combining multiple checks into a single statement. This capability is quite handy for scripting detailed decision trees in web development scenarios or any application logic.
What is short-circuit evaluation in JavaScript?
Short-circuit evaluation refers to the process by which JavaScript evaluates logical expressions. In the case of AND, it stops as soon as one false condition is encountered. For OR, evaluation ceases with the first true condition, optimizing performance by avoiding unnecessary checks.
How does one use logical operators for conditionals in JavaScript?
Logical operators are central to forming the conditions in if-else statements. They help determine the paths a program will take, integrating seamlessly with JavaScript’s control flow structures, impacting user experience based on dynamic conditions.
What are the common pitfalls when using JavaScript Logical Operators?
A frequent oversight is the misuse of == (equal to) instead of === (strictly equal to), not accounting for JavaScript’s type coercion, leading to unexpected bugs. Always understanding the data types you’re dealing with can prevent such errors.
Are logical operators only applicable to Boolean values?
While primarily used with Boolean values, JavaScript’s flexibility allows logical operators to be used with non-Boolean values as well. Through truthy and falsy evaluation, these operators work with various data types, contributing to the dynamic nature of web scripting.
How do Logical Operators interact with JavaScript’s falsy values?
JavaScript treats values like 0, “”, null, undefined, NaN, and false as falsy. Using logical operators with these can lead to surprising results if not handled carefully. This points to the necessity of understanding data types and conditional checking in software development.
How can logical operators enhance a JavaScript function?
Incorporating logical operators inside functions can streamline complex condition checks, allowing for concise and more readable code. They are instrumental in handling multiple parameter validations and enhancing the decision-making efficiency within functions.
Do newer JavaScript updates influence how logical operators work?
While the core functionality of logical operators remains intact, newer JavaScript features like optional chaining (?.) and nullish coalescing (??), offer more refined ways of handling undefined or null values, complementing the traditional use of logical operators, and simplifying some common coding scenarios.
Conclusion
In the landscape of web development, JavaScript Logical Operators like AND, OR, and NOT are indispensable. These tools not only shape the flow of decision-making within applications but also enhance user interactions by allowing dynamic responses to diverse conditions. From enhancing security checks to enriching user interfaces with conditional content, logical operators empower developers to write versatile, efficient, and maintainable code. Mastering their use is key to developing robust solutions that are both responsive and intuitive, making them a fundamental element in any developer’s toolkit.
- Tune In: Music Streaming Apps Like Pandora - June 20, 2024
- A Career as a Software Engineer: Pathways and Opportunities - June 20, 2024
- How to Create a User-Friendly Website - June 20, 2024