Adding Elements Efficiently: Javascript Array.push() Method
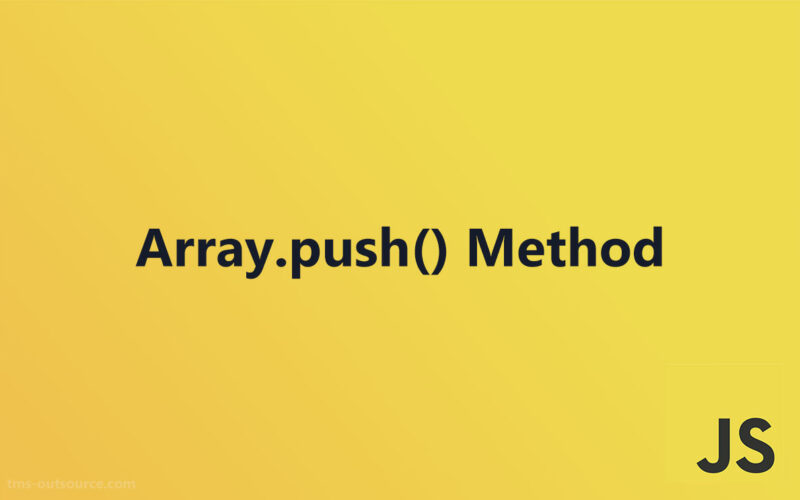
The Javascript Array.push() method is a powerful tool for web developers looking to manipulate arrays dynamically.
This method allows you to add new elements to the end of an array, modifying the original array in place.
By exploring its usage, benefits, and nuances, we can enhance array handling and optimize performance in web applications.
Detailed Examination of push() Syntax and Parameters
Syntax Breakdown

When working with arrays in JavaScript, understanding the syntax of the Javascript Array.push() Method can significantly enhance your coding practices. The general syntax form for this method is straightforward:
array.push(element1, element2, ..., elementN)
This method allows you to add one or more elements to the end of an array, directly modifying the original array rather than creating a new one.
Exploring variations and overloading examples, it’s intriguing how versatile this method is. Typically, you might use push()
to add elements of similar data types, but JavaScript allows for flexibility. For example, you can also push objects, functions, or even another array, which leads to different behaviors like nesting arrays within arrays.
Parameters Explained
Understanding the types of acceptable parameters is crucial. The push()
method can accept any type of JavaScript value—numbers, strings, objects, and even other arrays.
This flexibility makes it an invaluable tool in web development for managing array content dynamically.
The impact of different parameter types on array behavior is an important aspect. Adding simple data types like strings or numbers extends the array length appropriately and modifies the array content instantly.
However, pushing objects or arrays can introduce complexities. For example, if you push an array into another array, it adds the entire array as a single element, creating a nested array structure, which affects how you access these elements later in your operations.
Handling multiple elements and understanding the parameter’s influence on array performance and behavior allows developers to write more efficient and effective code, optimizing interactions within web applications.
Functional Description of push()
Operational Mechanism
Understanding how the Javascript Array.push() Method modifies an array is pivotal for effective array management in script writing.
Primarily, this method appends one or more elements to the end of an array and changes the original array, rather than creating a new one. Each element passed as a parameter to the push()
function is added to the array in the order they appear.
The significance of the return values of push()
cannot be understated. This method returns the new length of the array after the elements have been added.
This return value is particularly useful for immediate checks on array size, or for debugging purposes during development, ensuring that the array manipulation was successful.
Comparison with Similar Array Methods
When comparing push()
with similar array methods like pop()
, shift()
, and unshift()
, each serves a unique purpose in array manipulation.
While push()
adds elements to the end of an array, pop()
removes the last element from the array. In contrast, shift()
removes the first element, and unshift()
adds one or more elements to the beginning of the array.
Choosing when to use push()
versus other modifying methods depends on the specific needs of the application. If the requirement is to add elements to the end of an array and possibly track the array’s new length instantly, push()
is the optimal choice.
Conversely, in scenarios where you need to manipulate the beginning of the array or remove elements, using shift()
or pop()
would be more appropriate.
This decision deeply impacts the logic flow and performance of scripts, especially with larger data sets or performance-sensitive applications.
Practical Examples and Use Cases
Basic Usage Scenarios
At its core, the Javascript Array.push() Method is incredibly straightforward, making it a go-to tool in everyday coding projects. Its primary function is to add elements to the end of an array. Let’s break down how this plays out in common situations:
- Adding a single element: Simple enough, if you have an array like
let fruits = ['apple', 'banana']
, executingfruits.push('orange')
directly appends ‘orange’ to the end, mutating the original fruits array to['apple', 'banana', 'orange']
. - Adding multiple elements simultaneously: This method shines with its versatility, allowing multiple elements to be added at once. For example, when working with the same fruits array, one could execute
fruits.push('mango', 'papaya')
. Consequently, the array evolves to['apple', 'banana', 'orange', 'mango', 'papaya']
. This is particularly useful for dynamic array operations where the scope of data isn’t fixed.
Advanced Use Cases
For those who frequently engage with more complex coding scenarios, push()
also offers robust solutions:
- Merging two arrays with push(): Often in web development, merging arrays without creating a new array is necessary. Utilizing the
push()
combined with the...
spread operator achieves this seamlessly. Suppose there is an additional arraylet vegetables = ['carrot', 'potato'];
, merging it with the fruits array could be as simple asfruits.push(...vegetables)
, which updates fruits to['apple', 'banana', 'orange', 'mango', 'papaya', 'carrot', 'potato']
. - Using push() with call/apply for non-array objects: Despite being an array method,
push()
can be cleverly adapted for objects resembling arrays — objects with numerical keys and alength
property. Consider an object mimicking an array:let arrayLike = {0: 'hello', 1: 'world', length: 2};
. UsingArray.prototype.push.call(arrayLike, 'again')
effectively adds the string ‘again’ to this object, changing it to{0: 'hello', 1: 'world', 2: 'again', length: 3}
. This technique opens up new dimensions in functional programming and object manipulation.
Implementation of push() in Code
Step-by-step Coding Examples
Working with arrays is a fundamental skill in JavaScript, and the Javascript Array.push() Method provides a straightforward way to add elements, enhancing the dynamic capabilities of your applications.
- Pushing elements to an empty array: Consider an initial empty array where you need to start adding elements based on user input or data fetched from a server. Starting with an empty array, like
let numbers = [];
, you can easily add elements usingpush()
. For instance,numbers.push(1);
will change the array to[1]
. If another element, say2
, is added in similar fashion, the array updates to[1, 2]
. - Dynamic element addition in a loop: This approach is especially useful when dealing with multiple items, possibly coming from an API or user-generated content. Say you have a list of items
let itemsToAdd = ['apple', 'banana', 'cherry'];
, to add each to the arraylet fruits = [];
you could use a simple loop:for (let item of itemsToAdd) { fruits.push(item); }
Debugging Common Issues
As always, while the implementation might seem straightforward, certain complexities could arise during real-world applications, requiring a deeper understanding of array behaviors, especially in web development scenarios.
- Handling sparse arrays: Suppose there is an intention to create an array with non-sequential elements. Using
push()
carelessly might lead to unintended sparse arrays, which are arrays with empty slots. For example, creating an array and adding elements at non-consecutive indices directly could lead to performance hits since JavaScript engines handle sparse arrays less efficiently than dense ones. - Effects on array length and performance considerations: The efficiency of
push()
in terms of runtime can usually be high. However, repeatedly callingpush()
within high-frequency operations might impact performance, especially on large arrays or in environments where memory usage is a concern. Monitoring thelength
property before and after usingpush()
provides insights into how the method impacts the overall data structure, especially in applications where performance and memory footprint are critical.
push() Method Under the Hood
Technical Insights
Diving into how JavaScript engines handle array mutations reveals fascinating details about the efficiency and intelligence behind the scenes, especially concerning the Javascript Array.push() Method.
- How JavaScript engines handle array mutations: When an element is pushed into an array, the JavaScript engine optimizes the operation by adjusting the internal data structures that represent the array. These engines, such as V8 used in Google Chrome, maintain elements in contiguous memory locations as much as possible to optimize access and modification speed. However, if arrays become sparse due to deletions or large indexing, the engine switches to a different storage mechanism, which might reduce the performance.
- Memory management for growing arrays: Adding elements continuously with
push()
requires the JavaScript engine to allocate more memory to accommodate the growing array sizes. The engine handles this gracefully by initially allocating arrays with more capacity than currently needed to minimize the need for frequent reallocations. As arrays grow, the engines undertake periodic reallocations while maintaining operational efficiency.
Browser and ECMAScript Specifications
Understanding support across different environments ensures web applications function uniformly regardless of the user’s browser or the JavaScript version they support.
- Browser support for push(): This method is widely supported across all modern browsers, including Google Chrome, Firefox, Safari, and Edge. This universal support stems from the method’s inclusion in the earliest ECMAScript standards, making it a reliable tool in web development for array manipulation.
- ECMA specifications and compliance: The functionality of
push()
is well-defined in the ECMAScript standard, ensuring consistent behavior across different JavaScript implementations. The method complies with ECMAScript 5.1 and newer versions, which detail the algorithmic steps involved when an element is pushed onto an array, ensuring standardized performance and behavior in various execution contexts.
Best Practices and Performance Optimization
Optimizing Usage
While the Javascript Array.push() Method is typically efficient and straightforward, there are scenarios in large-scale applications where its usage might become suboptimal.
- When to avoid push() in large-scale applications: In cases where you’re dealing with extremely large arrays or performance-critical applications, consistently using
push()
can lead to memory inefficiency. This inefficiency arises because eachpush()
might cause a reallocation of the array to accommodate new elements, thereby temporarily doubling the memory footprint of the array. - Alternative methods and patterns for better performance: Instead of repeatedly using
push()
in a large-scale application, consider using better-performing alternatives like allocating an array with a predefined size or using data structures optimized for frequent additions and deletions, such as linked lists. If arrays are the only option, pre-allocating an array large enough to hold all the anticipated elements or usingconcat()
to merge arrays in a single operation can mitigate some ofpush()
‘s performance drawbacks.
Code Maintainability and Readability
Ensuring that the code remains maintainable and easy to read, especially when using array manipulation methods, is crucial.
- Writing readable code with push(): To maintain readability, avoid using
push()
inside complex expressions or deeply nested code structures. Instead, aim for clarity by keepingpush()
operations near the surface of your code logic, accompanied by comments that explain why elements are being added to the array. - Refactoring tips for legacy code using push(): When dealing with legacy code that heavily uses
push()
, consider gradually refactoring towards using more modern JavaScript features like spread syntax for combining arrays or destructuring assignments for more readable array manipulations. This approach not only clarifies what the code is intended to do but often leads to performance improvements by leveraging more efficient under-the-hood optimizations present in modern JavaScript engines.
FAQ On the JavaScript Array.push() Method
What exactly does the Javascript Array.push() Method do?
The Javascript Array.push() Method adds one or more elements to the end of an array and returns the new length of the array. This method modifies the original array directly, making it a staple in dynamic array manipulation scenarios in web development.
Can Array.push() accept multiple elements at once?
Yes, Array.push() can indeed handle multiple elements in a single call. You simply separate each element by a comma within the parentheses. For example, arr.push('apple', 'banana', 'cherry')
would add all three fruit names to the end of the arr
array.
Does Array.push() modify the original array or return a new one?
Array.push() modifies the original array. It directly adds the new elements to the existing array and does not create or return a new array. This in-place modification is crucial for handling data efficiently in many coding situations.
What is the return value of Array.push()?
Array.push() returns the new length of the array after the additional elements have been included. For instance, if three new items are pushed to an initially empty array, the method will return 3
.
Can you use Array.push() for arrays of objects?
Absolutely! Array.push() works seamlessly with arrays containing any data type, including objects. You can push new objects into the array or add properties to existing objects within the array, making it extremely versatile for object management.
How does Array.push() handle different data types?
Array.push() is data type-agnostic. Whether you’re working with strings, numbers, arrays, booleans, or objects, the method will effectively add the items to the end of the target array. This flexibility makes it invaluable for dynamic data handling in scripts.
What are the limitations of using Array.push() method?
While versatile, Array.push() can impact performance if used extensively on very large arrays due to needing to resize the array with each push. For massive datasets or performance-critical apps, alternative data structures or methods might be preferred to maintain efficiency.
How does Array.push() compare with other array methods like unshift()?
While Array.push() adds elements to the end of an array, Array.unshift() inserts elements at the beginning. Choosing between them depends on the specific requirements of how and where you want to add new data points in the array structure.
Is there a maximum number of elements you can push into an array?
Theoretically, the upper limit is determined by JavaScript’s maximum array length, which is about 2^32 – 1 elements. Practically, the limit could be impacted by available memory and performance considerations, especially in browser environments.
How do you handle errors with Array.push()?
Errors with Array.push() are rare, as it doesn’t typically fail on adding elements unless the array is somehow frozen or its prototype has been altered. Nonetheless, always ensure that the item being pushed is defined and the array is modifiable.
Conclusion
Throughout our exploration of the Javascript Array.push() Method, we’ve uncovered its robust functionality and versatility in array manipulation.
Whether you’re adding a single element or merging multiple arrays, push()
efficiently adjusts the size of the original array and enhances data management within your applications.
As we’ve seen, understanding the nuances of push()
, from its handling of various data types to its implications on memory management and performance, is crucial for any developer aiming to write optimized and maintainable code.
Embrace these insights to elevate your array handling strategies using this indispensible JavaScript method.
- Removing the Last Element with JavaScript Array.pop() Method - July 4, 2024
- Indoor Positioning Technology Explained: How It Works and Its Applications - July 4, 2024
- Laugh Out Loud: Comedy Apps Like iFunny - July 3, 2024