How to Use JavaScript Array delete Method Effectively
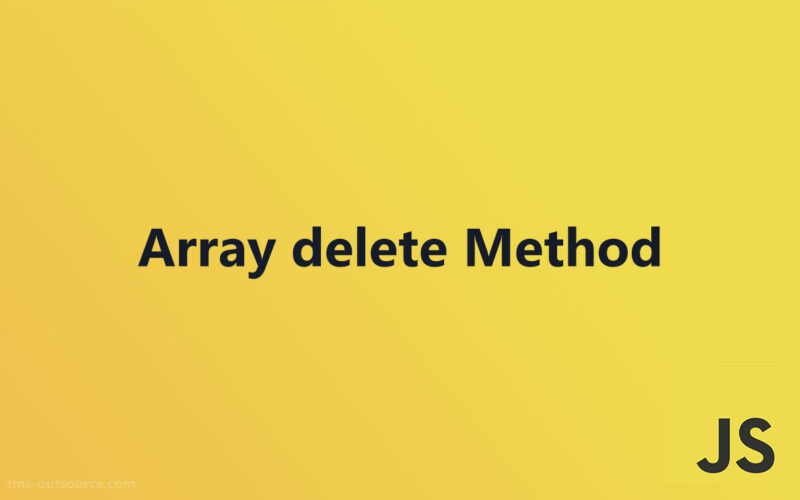
Arrays in JavaScript are dynamic collections of elements that are pivotal in handling data during the development process.
Understanding how to manipulate these arrays, particularly using the JavaScript Array delete Method, enhances functionality in your applications, allowing for efficient memory management and optimal data manipulation.
This method, among others, is critical for performing deletion operations variably to suit diverse programming scenarios.
Basic Array Methods
Methods for Adding and Removing Items
When working with arrays in JavaScript, understanding how to efficiently add and remove items is crucial for dynamic data manipulation and memory management.
Let’s dive into some fundamental methods provided natively in JavaScript.
Push() and Pop() – Adding and Removing at the End
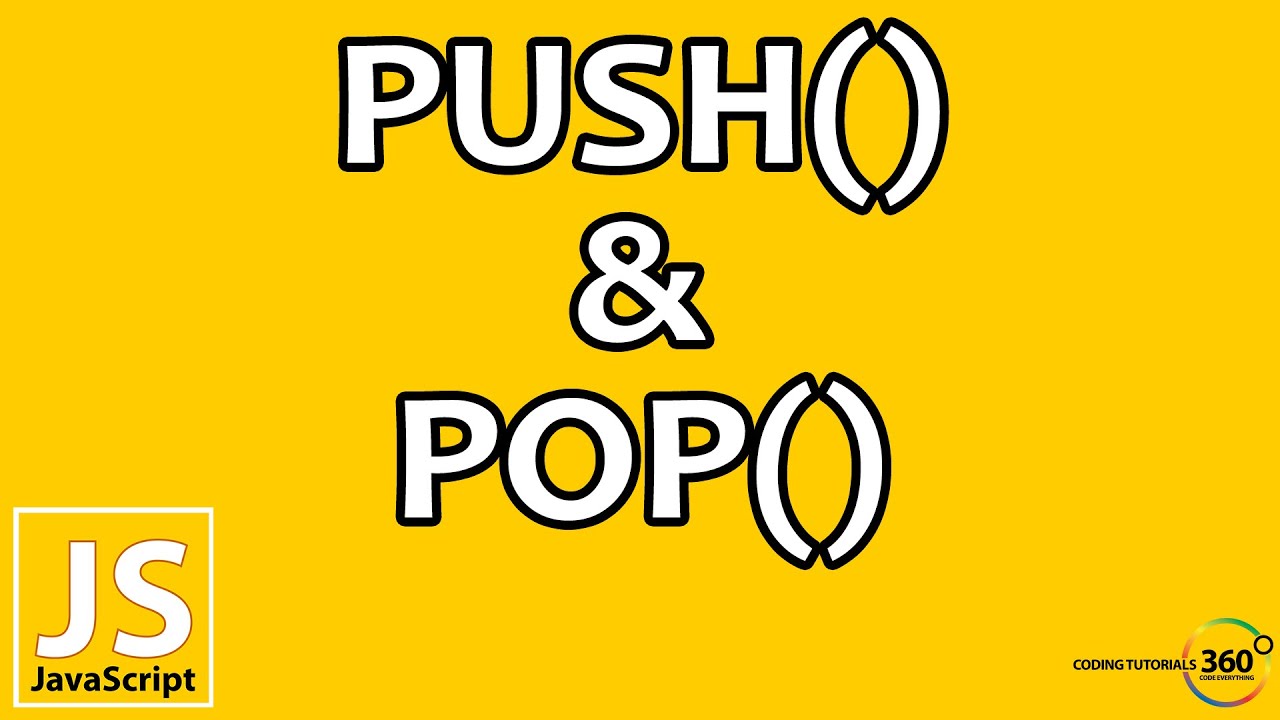
The push()
method allows you to add one or more elements to the end of an array, expanding it accordingly. This method is straightforward and one of the most frequently used due to its simplicity and direct action.
Conversely, pop()
is used to remove the last element from an array. This not only decreases the array’s length by one but also returns the removed element, which can be useful in many practical scenarios. For instance, managing stacks or last-in, first-out queues in data structures can benefit greatly from these methods.
Shift() and Unshift() – Adding and Removing at the Beginning
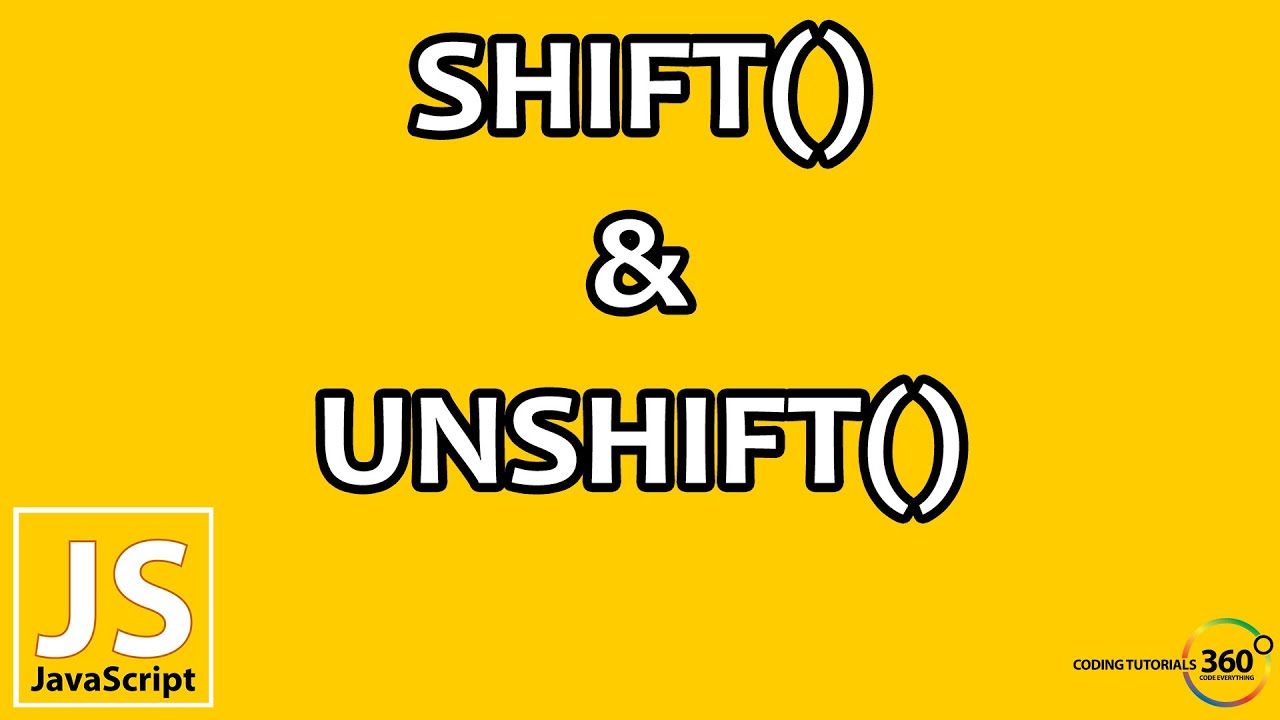
On the other hand, if you need to manipulate the beginning of an array, shift()
and unshift()
are your go-to methods. shift()
removes the first element of the array and also returns it. This method shifts the remaining elements to a lower index, which might affect performance in large arrays due to the re-indexing process.
unshift()
is like push()
, but adds elements to the beginning of the array. While extremely useful, just like shift()
, it can lead to slight performance issues with large arrays as it requires re-indexing the entire array.
Transformation and Representation Methods
Transforming and representing the data stored in arrays can be as critical as manipulating the arrays themselves. These methods ensure your data is in the correct format or structure for further processing or output.
toString() and join() – Converting Arrays to String Format
The toString()
method converts an array and its elements into a string, separating the elements with commas.
This is particularly handy when you need a quick conversion of array data into a readable string format without much fuss.
join()
takes this a step further by allowing you to specify a separator. For example, join(' - ')
would convert the array elements into a string with elements separated by dashes.
This method is essential for creating neatly formatted outputs from array contents, adhering to specific requirements of web development JavaScript arrays or even just improving JavaScript array tutorials.
slice() and splice() – Modifying Array by Removal or Replacement
slice()
is a method that returns a shallow copy of a portion of an array into a new array object. It is non-destructive, meaning the original array will not be modified.
This method is incredibly flexible, as it allows you to specify start and end indices for the slice you need. It is often used for situations where you need subsets of existing arrays without altering the original array.
splice()
, however, can fundamentally change an array by adding, removing, or replacing elements.
It is a powerful tool in array manipulation, providing the means to directly modify the content of an array starting from any index.
Practical examples of splice()
demonstrate its versatility, but they also highlight the need for careful implementation to avoid unintended side effects in your data structure.
Both slice()
and splice()
are pivotal when working with arrays in JavaScript, offering control over array data with precision.
Whether it’s through extracting elements using slice()
or modifying content directly with splice()
, these methods are fundamental in effective JavaScript coding and play a significant role in managing array length and performance.
Advanced Array Manipulation Techniques
Using splice() for Removal and Addition
Manipulating arrays effectively requires a deeper understanding of available methods that go beyond basic addition and removal. splice()
is a versatile JavaScript function that allows for robust array manipulation, including both removal and addition of items within a single operation.
Syntax and Parameters of splice()
splice()
method can be somewhat intimidating due to its versatility. The basic syntax of splice()
is:
array.splice(start[, deleteCount[, item1[, item2[, ...]]]])
- start: The index at which to start changing the array.
- deleteCount (optional): The number of elements to remove from the array.
- item1, item2, … (optional): Elements to add to the array, starting from the
start
index.
Understanding each parameter is vital for implementing splice()
correctly. The ability to simultaneously remove and add elements makes splice()
a powerful tool for array editing.
Practical Examples of splice() in Use
Consider you need to replace certain elements within an array. Instead of using separate operations to remove and then add elements, splice()
can handle both in one go. Here’s a brief example:
let fruits = ["apple", "banana", "cherry", "date"];
fruits.splice(2, 1, "orange", "grape"); // Replaces 'cherry' with 'orange' and 'grape'
In this use case, splice()
starts at index 2, removes one element (‘cherry’), and adds both ‘orange’ and ‘grape’ in its place. The result is a clear demonstration of splice()
‘s utility in manipulating array contents directly, making it an indispensable method for web developers dealing with JavaScript arrays.
The delete Operator
While splice()
is tailored for direct manipulation within arrays, the JavaScript Array delete Method offers a different approach, ideal for removing elements without affecting the length of the array.
Characteristics and Limitations of delete
The delete
operator is used to remove properties from objects. It’s important to understand that using delete
on an array element does not affect the array’s length. Instead, it leaves a hole in the array, setting the element at the specified index to undefined
.
let numbers = [1, 2, 3, 4];
delete numbers[2]; // numbers becomes [1, 2, undefined, 4]
This characteristic is crucial to remember because it can lead to unexpected issues in array processing, where undefined
values might not be handled as expected.
Implications of Using delete on Array Properties
Using delete
on array properties is not generally recommended for array manipulation due to its side effect of leaving undefined
holes. This becomes problematic in operations expecting contiguous items, such as when mapping over arrays or converting arrays to strings.
In scenarios where maintaining the integrity of array indices is not critical, or handling undefined
entries is accounted for in your logic, delete
can be a useful operator. However, understanding its limitations and effects is essential for proper implementation.
In practical terms, these methods each have their place in JavaScript development, dependent on the specific requirements and contexts of your projects. Assessing when to use splice
versus delete
can mark the difference between efficient data handling and potential performance pitfalls.
Functional Programming Methods
Utilizing filter() for Conditional Removals
Understanding how filter()
works is integral to manipulating arrays in JavaScript, especially when you need to remove elements based on specific conditions without altering the original array.
Definition and Use of the filter() Method
The filter()
method creates a new array with all elements that pass the test implemented by the provided function. It’s the perfect tool for situations where you need to sift through data and extract elements that meet certain criteria.
const numbers = [1, 2, 3, 4, 5];
const evenNumbers = numbers.filter(number => number % 2 === 0);
In the example above, filter()
checks each element against the condition number % 2 === 0
, which effectively selects even numbers. This seamless method of conditional removal keeps your original array intact, offering both functionality and safety in array manipulation.
Examples Demonstrating filter() in Action
To further illustrate, consider a scenario where you have an array of users and need to filter out those who are not active:
const users = [
{ name: "Alice", active: true },
{ name: "Bob", active: false },
{ name: "Carol", active: true }
];
const activeUsers = users.filter(user => user.active);
This code snippet efficiently isolates active users, underscoring filter()
‘s utility in handling arrays based on dynamic conditions. Its application extends beyond simple checks to more complex data filtration tasks in web development.
Combination of indexOf() and splice()
For direct manipulation by removing elements at specific positions, combining indexOf()
and splice()
provides a precise solution.
Finding Element Positions with indexOf()
When you need to find the first occurrence of a specific element in an array, indexOf()
is the method to use. It returns the index of the element, or -1 if the element is not found.
const pets = ["cat", "dog", "bird", "fish"];
const birdIndex = pets.indexOf("bird"); // returns 2
Knowing the index allows for targeted manipulation, which is where splice()
comes into play.
Removing Items by Index with splice()
Once you have the index of the element you wish to remove, splice()
can be used to effectively remove it from the array.
if (birdIndex !== -1) {
pets.splice(birdIndex, 1); // removes 'bird'
}
This combination of indexOf()
and splice()
is particularly powerful for removing items when only the value is known, not the position. It offers precision in array editing, essential for maintaining data integrity and performance when working with JavaScript arrays. The efficiency of this approach also adheres to JavaScript programming best practices, ensuring that your code remains both maintainable and functional.
Third-Party Library Methods
Lodash Library for Enhanced Array Manipulation
Delving into third-party libraries can significantly expand the functionality and ease of array manipulations in JavaScript. Lodash is a standout library known for its consistency, performance, and additional features that go beyond native JavaScript capabilities.
Introduction to Lodash and its Utilities
Lodash is a JavaScript utility library that simplifies the process of working with arrays, numbers, objects, strings, etc.
It’s particularly revered for its rich collection of methods that help programmers write more concise and maintainable JavaScript code. Lodash’s methods are robust, handle edge cases well, and are optimized for performance.
For array manipulations, Lodash offers a variety of tools that streamline operations which might otherwise require complex and verbose code. This simplification comes without sacrificing speed or capability, effectively boosting productivity and ensuring code efficiency.
_.remove() Method for Condition-Based Element Removal
Among the plethora of useful methods in Lodash, _.remove()
is particularly valuable for condition-based modifications of arrays. This method mutates the array by removing elements that satisfy a condition specified in the callback function, filling a gap that the native JavaScript Array delete Method does not directly address.
const numbers = [1, 2, 3, 4, 5, 6];
_.remove(numbers, function(n) {
return n % 2 === 0;
});
In this example, _.remove()
takes an array of numbers and a callback function that determines the removal condition (in this case, removing even numbers).
The method directly modifies the original array, providing a straightforward and powerful way to dynamically adjust array contents based on complex criteria.
The use of Lodash, especially methods like _.remove()
, epitomizes the advantages of integrating well-maintained and community-trusted third-party libraries into web development projects.
These tools not only extend the capabilities of JavaScript but also encapsulate common coding tasks into simpler, more readable operations, aligning with best practices for modern JavaScript development.
Third-Party Library Methods
Lodash Library for Enhanced Array Manipulation
Exploring third-party libraries like Lodash can revolutionize the way you handle arrays in JavaScript.
Lodash, in particular, is celebrated for streamlining complex tasks through its comprehensive suite of utilities that optimize both time and readability in coding.
Introduction to Lodash and its Utilities
Lodash is a standout tool that makes JavaScript easier by taking the hassle out of working with arrays, numbers, objects, and strings.
Unlike the basic JavaScript methods, Lodash brings consistency to operations across browsers and contributes significantly to code readability and maintenance.
It is meticulously optimized for performance and offers a more functional programming approach.
The library includes a wealth of utilities designed to enhance coding efficiency and simplify tasks that would otherwise be cumbersome when using native JavaScript methods alone. It is particularly useful in projects where the manipulation of data collections is frequent and complex.
_.remove() Method for Condition-Based Element Removal
One of the powerful methods Lodash offers is _.remove()
. This function goes beyond what the JavaScript Array delete Method can achieve by allowing conditional removal of items directly from the original array.
// Sample array of objects
const users = [
{id: 1, name: 'John', active: true},
{id: 2, name: 'Jane', inactive: false}
];
// Conditionally remove inactive users
_.remove(users, {inactive: false});
This example demonstrates how _.remove()
can efficiently filter out elements without leaving undefined spaces or requiring additional steps to handle array re-indexing.
The method mutates the array based on the condition provided, which in this case removes any user object where the inactive property is false.
Lodash’s _.remove()
strategically combines simplicity with powerful capabilities, enabling a cleaner and more effective approach to array manipulation.
By integrating such third-party libraries, developers can focus more on the innovative aspects of their projects, leveraging ready-made functionalities for better productivity and robust performance in their applications.
Comprehensive Examples and Use Cases
Removing Elements by Value
In the realm of web development, efficiently managing data within arrays is a common task. Removing elements by their values demonstrates a practical application of array manipulation methods.
Detailed Use Case: Identifying and Removing Specific Elements
Consider a scenario where you need to remove specific elements from an array based on their value. For example, let’s remove all occurrences of a specific value from an array of integers.
let numbers = [1, 2, 3, 4, 2, 2, 5];
numbers = numbers.filter(number => number !== 2);
This code snippet uses the filter()
method to create a new array excluding the value 2. This approach is not only straightforward but also leaves the original array unmodified, which is a best practice in scenarios where the original data integrity is crucial.
Comparing Performance: Native Methods vs. Lodash Implementation
To understand the efficiency of native JavaScript methods versus Lodash implementations, let’s compare how each handles removing elements.
Using native JavaScript, filter()
method is both clean and effective for not modifying the original array.
Lodash’s _.remove()
function, on the other hand, modifies the array in place, which can be more memory efficient in large-scale applications due to reducing the need to create a copy of the array.
Performance-wise, native methods may be preferable for smaller or less complex datasets where simplicity and readability are prioritized.
Lodash may offer better performance in intensive applications, especially those needing high optimization and complex array manipulations.
Best Practices for Code Clarity and Efficiency
Cultivating best practices for array manipulation ensures code clarity and computational efficiency, which are paramount to maintaining scalable and manageable codebases.
When to Use Native JavaScript Methods Over Third-Party Libraries
Native JavaScript methods should be your first consideration for array manipulations because they typically do not require additional dependencies and adhere to JavaScript standards.
Use third-party libraries like Lodash when dealing with high complexity problems where specialized methods greatly reduce the time and code needed to implement solutions.
Tips for Writing Maintainable and Efficient JavaScript Code
To write maintainable and efficient code:
- Always prefer immutable methods like
map()
,filter()
, orreduce()
over methods that modify the array directly. - Use clear and descriptive variable names.
- Break down large functions into smaller, reusable functions.
- Regularly review and refactor code to improve readability and performance.
- Leverage modern JavaScript ES6+ features for more concise and optimized code, such as arrow functions, spread syntax, and destructuring.
Integrating these practices will not only improve the effectiveness of your array manipulations but also enhance your overall approach to writing JavaScript code, ensuring that it’s both powerful and easy to understand.
FAQ On JavaScript Array Delete Method
What exactly does the JavaScript Array delete Method do?
The delete
method in JavaScript specifically targets elements within an array, setting them to undefined
. However, crucially, it does not alter the array’s length, leaving a gap where the deleted element was positioned.
What’s the major downside of using delete in JavaScript arrays?
Using delete
creates empty slots in the array, referred to as “holes.” These undefined spots can lead to unexpected behavior during array traversal and operations, as they just become empty values without adjusting the size of the array.
How does delete compare with the splice method for removing array elements?
Although both can remove elements, splice
is generally more versatile. Unlike delete
, splice
removes the element and adjusts the array’s length, preventing any undefined slots and keeping the array compact.
Is the delete method able to completely remove an element from memory?
No, delete
doesn’t fully reclaim memory if other references to the same item exist. It sets the specific index to undefined
but any references to the deleted element through other variables will remain intact in memory.
Can you use delete on an array that you’ve declared with const?
Yes, you can. While const
prevents reassignment of the variable, it doesn’t make the array immutable. You can still modify the contents of the array, including using delete
to set elements to undefined
.
What happens to array length after using the delete method?
The length of the array remains unchanged after using delete
. This method only clears the value at the specified index, turning it into undefined
without altering the physical size of the array.
How can you find and remove specific elements without leaving holes in an array?
The best approach is using filter()
or splice()
. filter()
creates a new array excluding unwanted elements, while splice()
can directly remove items and shift the remaining elements to fill the gap.
Are there any performance concerns with using delete?
Yes, particularly in cases where you are managing large arrays. Since delete
leaves holes, subsequent operations that skip these undefined indices can lead to decreased performance and unexpected bugs in complex applications.
What is a better alternative to delete for handling deletion of multiple array elements?
For multi-element removal, splice()
is more effective. It allows for the deletion of several contiguous elements within a single operation and automatically adjusts the array’s size, which maintains clean and compact data structures.
In what scenario might you still consider using delete with an array?
In situations where the array’s length must remain unchanged, or you specifically need to preserve the indices of remaining elements, using delete
becomes a viable option. It’s beneficial when you need to maintain placeholder values within an array structure.
Conclusion
In essence, the JavaScript Array delete Method plays a unique role in array manipulation, specifically setting elements to undefined
without changing the array’s length. While it provides an avenue for modifying arrays without restructuring their order, alternatives like splice()
and filter()
often offer more practical solutions by avoiding the pitfalls of empty slots. Understanding when and how to use these methods can significantly enhance your coding efficiency, especially in situations where performance and clarity are paramount. Each method brings its own strengths, making JavaScript a versatile tool in managing data within applications.
- Stream and Game: Engaging Apps Like Twitch - July 1, 2024
- Flattening Nested Arrays with JavaScript Array flat() Method - July 1, 2024
- Create and Share: Fun Video Apps Like Dubsmash - June 30, 2024