Combining Arrays with JavaScript Array concat() Method
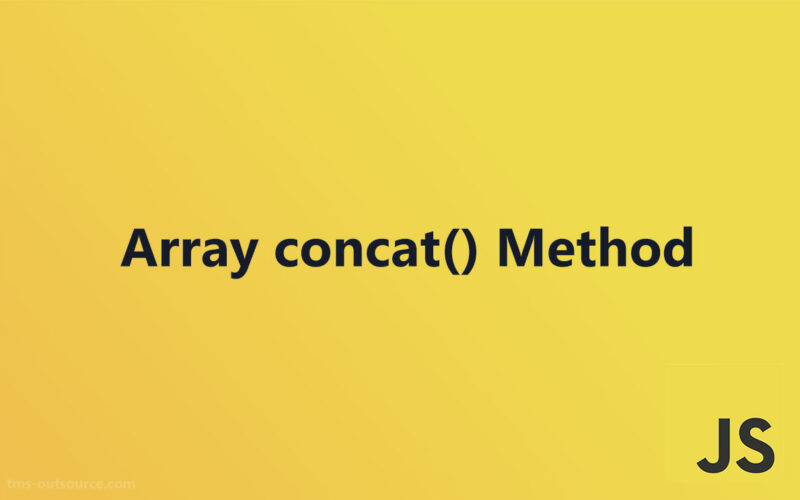
The JavaScript Array concat() Method is a powerful tool in web development for merging two or more arrays.
This method does not modify the existing arrays but instead returns a new array, making it indispensable for managing state in JavaScript frameworks and ensuring data immutability.
Understanding its syntax, parameters, and practical applications enhances efficiency and effectiveness in coding practices.
Syntax and Parameters
Syntax of the concat() Method

When diving into the syntax of the concat() method, it’s crucial to grasp its straightforward nature.
The general syntax appears as follows: you’d start with an existing array, tack on a period, and type “concat()”, with the arrays or values you want to merge passed inside the parentheses.
For example, the basic structure looks like this:
let newArray = firstArray.concat(secondArray);
While this is the most common usage, the JavaScript concat() Method accepts more than just single arrays.
The method supports multiple arguments simultaneously, including arrays, values, or a combination thereof. This flexibility means you can execute quite dynamic operations.
For web development tasks involving data manipulation, knowing these variations allows for efficient data handling and ensures you adhere to best practices in JavaScript programming.
Parameters Explained
Understanding the parameters accepted by concat() deepens your command of its capabilities.
This method is quite accommodating since it can handle various types of arguments. Primarily, these include:
- Single Arrays:Â This is the most straightforward use, where one array is concatenated to another.
- Multiple Arrays:Â You can pass multiple arrays within a single concat() call.
- Primitive Values:Â Integers, strings, and booleans can also be concatenated, directly appended to the resultant array.
Here are a couple of examples that showcase different argument types:
- Concatenating two arrays:
let fruits = ['apple', 'banana']; let vegetables = ['carrot', 'daikon']; let food = fruits.concat(vegetables);
- Adding elements directly:
let numbers = [1, 2]; let moreNumbers = numbers.concat(3, 4, [5, 6]); // moreNumbers is [1, 2, 3, 4, 5, 6]
- Combining arrays with objects and strings:
let items = [{ name: 'chair' }, { name: 'desk' }]; let moreItems = items.concat({ name: 'lamp' }, ['book', 'pen']);
In handling array-like objects and values with the Symbol.isConcatSpreadable property, the concat() method is influenced by whether this property’s set to true—to treat it like an array—or false.
These capabilities support robust applications like managing state in JavaScript frameworks, where combining datasets effectively can significantly streamline state management processes.
It also helps in dealing with advanced JavaScript array functions, optimizing both performance and readability of your code in various web development scenarios.
Return Values and Behavior
Understanding the Return Value
When using the JavaScript Array concat() Method, the return value is often straightforward but crucial to understand for effective data manipulation.
This method always returns a new array containing the original elements from the source array(s) plus any additional arguments added via the method. The key characteristics to note here are:
- New Array Creation:Â Concat() does not alter the existing arrays but rather produces a new array, ensuring the immutability of the original arrays.
- Shallow Copy:Â The method performs a shallow copy of the array elements. If an array contains objects, the references to those objects are copied (not the actual objects), which may impact the behavior when modifying these objects later.
- Type Handling:Â All data types are accepted, making this method versatile. If non-array arguments are specified, they are directly appended to the result array as new elements.
Here’s an illustrative example of how different data types are handled:
let numbers = [1, 2];
let moreNumbers = numbers.concat('3', [4, 5]);
// moreNumbers is [1, 2, '3', 4, 5]
Note the string ‘3’ and the numbers within the nested array are seamlessly integrated into the resultant array.
Behavior with Special Cases
While the concat() method typically behaves predictably, certain special cases require extra attention to avoid unintended results in your web development projects:
- Concatenation of Nested Arrays:Â When nested arrays are passed as arguments, concat() only shallow copies their references into the new array. These aren’t flattened by default unless the nested array has theÂ
Symbol.isConcatSpreadable
 property set to true. - Handling Sparse and Dense Arrays: If you concat sparse arrays (arrays with missing elements), the missing indexes are treated as undefined. This might lead to unexpected gaps in the resultant array.
- Edge Cases in Behavior:Â There are scenarios where the concat() might behave unexpectedly. For instance, when exceeding the JavaScript engine’s limit on the array’s length or manipulating large arrays, you might encounter performance hits or, in extreme cases, a stack overflow error.
Consider these examples:
let denseArray = ['a', 'b', 'c'];
let sparseArray = [];
sparseArray[5] = 'sparse';
let combined = denseArray.concat(sparseArray);
// combined is ['a', 'b', 'c', undefined, undefined, 'sparse']
The undefined entries represent gaps from the sparse array, showcasing how sparse arrays can influence the output.
Understanding these special behaviors helps in crafting robust, error-free JavaScript code, optimizing array manipulations while ensuring your applications manage data efficiently.
Practical Examples
Basic Usage
When it comes to understanding the JavaScript Array concat() Method, seeing it in action provides the clearest insight. Starting with the basics:
- Concatenating Two Arrays:Â Simply merge two arrays into one. This is particularly handy when you need a quick way to gather elements from different sources.
let fruits = ['apple', 'banana'];
let vegetables = ['carrot', 'beet'];
let combinedFood = fruits.concat(vegetables);
// combinedFood is ['apple', 'banana', 'carrot', 'beet']
- Concatenating Multiple Arrays:Â You can also blend more than two arrays at once, which simplifies handling larger datasets or combining multiple sources of data in one go.
let coldDrinks = ['soda', 'water'];
let hotDrinks = ['coffee', 'tea'];
let snacks = ['chips', 'pretzel'];
let partyMenu = coldDrinks.concat(hotDrinks, snacks);
// partyMenu is ['soda', 'water', 'coffee', 'tea', 'chips', 'pretzel']
Advanced Examples
As your scenarios grow in complexity, so does the utility of the concat() method:
- Concatenating Arrays with Objects:Â Merging arrays containing objects reveal the method’s handling of reference types. The objects are not duplicated but referenced, so changes to the original affect the concatenated array.
let objectArray1 = [{ item: 'pen' }, { item: 'pencil' }];
let objectArray2 = [{ item: 'eraser' }];
let stationery = objectArray1.concat(objectArray2);
// Changes to objects in original arrays reflect in 'stationery'
- Handling Array-like Objects and Symbol.isConcatSpreadable:Â This property allows finer control over how objects are treated when used with concat(). Setting it to true tells concat() to treat the object as an array.
let arrayLikeObject = { 0: 'book', 1: 'notebook', length: 2, [Symbol.isConcatSpreadable]: true };
let papers = ['paper'];
let officeSupplies = papers.concat(arrayLikeObject);
// officeSupplies is ['paper', 'book', 'notebook']
Real-world Applications
Beyond basic examples, the concat() method serves key functions in more complex applications:
- Data Manipulation in Web Development:Â It’s a staple when handling dynamic data arrays drawn from APIs or user inputs. Whether it’s combining user data from different sources or aggregating results from multiple queries, concat() makes these tasks more manageable.
- Use Cases in Handling State in JavaScript Frameworks:Â Modern JavaScript frameworks often rely on immutable patterns for state management. Using concat(), developers can efficiently manage state without mutating the original state, which is crucial for predictability and maintainability of the application state. For instance, in scenarios where you need to add new items to an array stored in the state of a React application, concat() can merge arrays without side effects.
Technical Insights
Performance Considerations
When using the JavaScript Array concat() Method, understanding its efficiency in various scenarios can help optimize your code significantly. The method is generally fast and reliable for smaller to medium-sized arrays but can be less efficient with very large arrays due to the need to create a new array and copy over elements from the original arrays.
In comparison, alternative methods like the spread syntax (...
) offer similar functionality with potentially better performance in handling large datasets. Here’s a quick look:
let array1 = [1, 2, 3];
let array2 = [4, 5, 6];
// Using concat()
let combinedUsingConcat = array1.concat(array2);
// Using spread syntax
let combinedUsingSpread = [...array1, ...array2];
Both approaches achieve the same result, but the spread syntax can be more succinct and, in some cases, faster, especially when dealing with complex or computational-heavy array operations. It’s crucial to assess which method suits your specific situation best, particularly in web development where efficiency can impact user experience.
Compatibility and Polyfills
Browser support is another vital consideration for developers leveraging modern JavaScript methods. The good news is, the concat() method enjoys widespread support across all major browsers, alleviating concerns about compatibility in standard use cases.
However, when using newer or more elaborate features associated with arrays, you might face limitations in older browsers. In such instances, implementing polyfills is a sensible approach. A polyfill allows you to reproduce the functionality of the concat() method in environments where it isn’t natively supported, ensuring your applications remain functional across a broad range of browsers.
if (!Array.prototype.concat) {
Array.prototype.concat = function() {
// Implementation of concat functionality
};
}
By incorporating such polyfills, you can extend compatibility to older browsers, maintaining the robustness and accessibility of your web applications across various user environments. This practice is part of ensuring that web development projects remain inclusive and functional irrespective of the user’s browser version.
FAQ On the JavaScript Array concat() Method
What exactly does the JavaScript Array concat() Method do?
The concat()
 method in JavaScript is used to merge two or more arrays into one. This method does not change the existing arrays but rather returns a newly formed array that includes elements from the input arrays.
Can you concatenate more than two arrays at once with concat()?
Absolutely! The concat()
 method can take multiple arrays as arguments. It merges them sequentially into a single new array, which simplifies handling various data sets efficiently.
Does the concat() method modify the original arrays?
No, it does not. The concat() method produces a new array while leaving the original arrays untouched. This behavior ensures data immutability, which is a crucial concept in modern JavaScript development.
How does concat() handle different data types within arrays?
concat()
 seamlessly integrates different data types. It can process strings, numbers, objects, and even nested arrays without issues, appending each element to the new array in the order they appear.
What happens if you use concat() with non-array parameters?
If non-array arguments are provided, concat()
 treats them as array elements and appends them directly to the resultant array. This flexibility allows for dynamic array creation and manipulation in various scripting scenarios.
How does the concat() method deal with sparse arrays?
When sparse arrays are concatenated, concat()
 treats missing elements as undefined. This can result in gaps in the new array, filled with undefined values proportional to the absent elements in the original sparse arrays.
Are there any performance considerations when using concat() on very large arrays?
Yes, while concat()
 is generally efficient, its performance might degrade with extremely large arrays because it involves creating a new array and copying elements. In such cases, other methods like the spread syntax can be more performant.
Is the concat() method compatible with all browsers?
concat()
 enjoys broad support across all modern web browsers. However, for older browsers that might not support it, developers can implement polyfills to extend its functionality to those environments.
What is the difference between using concat() and the spread syntax for merging arrays?
While both achieve similar outcomes, the spread syntax (...
) is often more succinct and can be faster than concat()
 in operations involving large datasets. Unlike concat, spread also allows easy insertion of elements at specified positions within the new array.
How do you handle objects and the Symbol.isConcatSpreadable property in concat()?
Objects in arrays are concatenated by reference. If an object has the Symbol.isConcatSpreadable
 property set to true, concat()
 treats it as an array, spreading its elements instead of adding the object as a single element.
Conclusion
The JavaScript Array concat() Method is an invaluable tool in the arsenal of any developer committed to crafting efficient, effective web applications.
Its ability to merge arrays seamlessly, while maintaining the immutability of the original data, allows for sophisticated data manipulation and state management.
Whether dealing with basic arrays or incorporating objects with the Symbol.isConcatSpreadable
 property, concat() handles it with finesse.
As web technologies evolve, understanding and utilizing this method efficiently can notably enhance the functionality and performance of your web development projects.
Embrace it to ensure your data handling is as seamless and effective as possible.
- Adding Elements Efficiently: Javascript Array.push() Method - July 2, 2024
- The Role of AI in IT Outsourcing - July 2, 2024
- Stream and Game: Engaging Apps Like Twitch - July 1, 2024